c怎么将字符串分割并存去数组
时间: 2024-05-12 09:17:47 浏览: 29
可以使用字符串函数和循环来实现字符串的分割和存储到数组中,具体实现过程如下:
1. 定义一个字符数组用于存储字符串,再定义一个整型变量用于记录字符串长度。
2. 使用字符串函数strlen()获取字符串长度。
3. 定义一个字符指针p,将其指向字符串的首地址。
4. 定义一个整型变量count,初始化为0,用于记录分割后的字符串数量。
5. 使用循环遍历字符串,遇到分隔符则将前面的字符串存储到一个新的字符数组中,将其作为一个元素存储到目标数组中。
6. 循环结束后,用count记录的数量作为返回值。
具体实现代码如下:
```
#include <stdio.h>
#include <string.h>
int split(char *str, char *delim, char **result)
{
int len = strlen(str);
char *p = str;
int count = 0;
while (*p)
{
if (*p == *delim)
{
*p = '\0';
result[count] = str;
count++;
str = p + 1;
}
p++;
}
if (str != p)
{
result[count] = str;
count++;
}
return count;
}
int main()
{
char str[] = "hello world!";
char *delim = " ";
char *result[10] = { 0 };
int count = split(str, delim, result);
for (int i = 0; i < count; ++i)
{
printf("%s\n", result[i]);
}
return 0;
}
```
输出结果为:
```
hello
world!
```
阅读全文
相关推荐
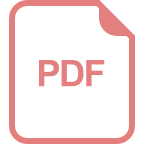
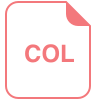
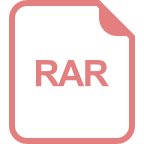
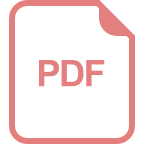
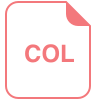
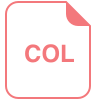


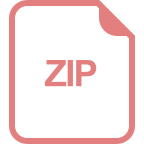
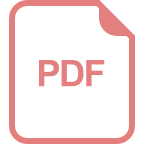
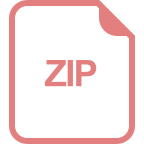
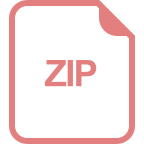
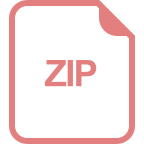