numpy多个数组级联
时间: 2024-11-06 13:13:18 浏览: 23
在NumPy库中,你可以使用`numpy.concatenate()`函数将多个数组沿着指定的维度连接在一起。这个函数可以水平地(column-wise)连接(堆叠)数组,也可以垂直地(row-wise)连接它们。基本语法如下:
```python
numpy.concatenate(arrays, axis=0)
```
其中,
- `arrays` 是你想连接的一组数组列表。
- `axis` (默认为0) 指定了连接的方向:
- 如果 `axis=0`(默认),数组会按行堆叠;
- 如果 `axis=1`,则按列堆叠。
例如:
```python
import numpy as np
# 创建两个一维数组
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
# 水平连接(按行)
result1 = np.concatenate((arr1, arr2))
print(result1)
# 垂直连接(按列)
result2 = np.concatenate((arr1[:, np.newaxis], arr2[:, np.newaxis]), axis=1)
print(result2)
```
阅读全文
相关推荐
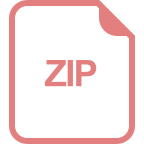
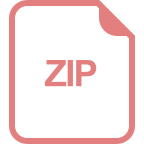
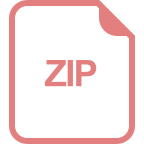
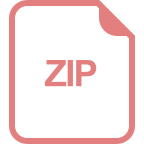
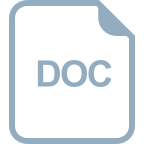
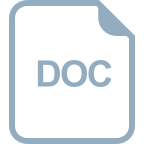
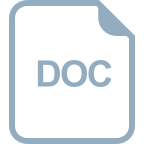
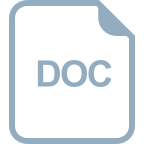
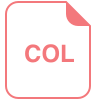
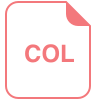
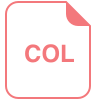
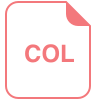
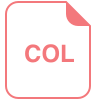
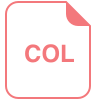
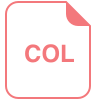
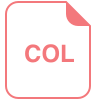
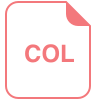
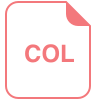
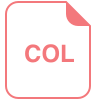