OpenCV图像特征提取:9个步骤,揭开图像背后的秘密
发布时间: 2024-08-13 21:43:31 阅读量: 23 订阅数: 32 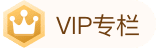
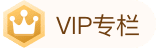

# 1. 图像特征提取概述**
图像特征提取是计算机视觉领域中至关重要的一步,它旨在从图像中提取具有代表性的信息,这些信息可以用来识别、分类和分析图像。图像特征可以是颜色、形状、纹理或其他视觉属性,它们可以帮助计算机理解图像的内容。
图像特征提取过程通常包括以下步骤:
1. **图像预处理:**对图像进行必要的处理,例如灰度化、噪声去除和图像增强。
2. **关键点检测:**识别图像中具有独特特征的点,这些点可以是角点、边缘点或其他感兴趣区域。
# 2. 图像特征提取基础
### 2.1 图像特征的类型和应用
图像特征是图像中能够描述其内容和性质的显著属性。它们可以分为以下几类:
- **全局特征:**描述图像的整体属性,例如颜色直方图、纹理特征和形状描述符。
- **局部特征:**描述图像局部区域的属性,例如关键点、边缘和角点。
图像特征在计算机视觉和图像处理中有着广泛的应用,包括:
- **图像分类:**将图像分类到不同的类别,例如动物、植物和风景。
- **对象检测:**在图像中定位和识别特定对象。
- **图像检索:**基于图像特征搜索和检索相似的图像。
- **图像配准:**将两幅或多幅图像对齐,以便进行比较和分析。
- **图像分割:**将图像分割成不同的区域或对象。
### 2.2 OpenCV中图像特征提取的原理
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,提供了广泛的图像特征提取算法。这些算法基于以下原理:
- **关键点检测:**识别图像中具有显著变化的区域,例如角点、边缘和斑点。
- **描述符提取:**计算关键点周围区域的特征向量,描述其外观和性质。
- **特征匹配:**比较不同图像中关键点的描述符,以找到匹配点。
OpenCV中常用的图像特征提取算法包括:
- **SIFT(尺度不变特征变换):**一种对尺度和旋转不变的局部特征。
- **SURF(加速稳健特征):**一种比SIFT更快的局部特征。
- **ORB(定向快速二进制模式):**一种计算效率高的局部特征。
- **HOG(方向梯度直方图):**一种用于检测和识别对象的部分全局特征。
**代码块:**
```python
import cv2
# 加载图像
image = cv2.imread('image.jpg')
# 使用SIFT算法检测关键点
keypoints = cv2.SIFT_create().detect(image)
# 绘制关键点
cv2.drawKeypoints(image, keypoints, image)
# 显示图像
cv2.imshow('Keypoints', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
这段代码使用OpenCV中的SIFT算法检测图像中的关键点。SIFT算法通过寻找图像中具有显著变化的区域来检测关键点。检测到的关键点以圆圈的形式绘制在图像上。
**参数说明:**
- `cv2.SIFT_create()`:创建SIFT算法对象。
- `detect()`:使用SIFT算法检测图像中的关键点。
- `drawKeypoints()`:将关键点绘制在图像上。
- `imshow()`:显示图像。
- `waitKey()`:等待用户按下键盘上的任意键。
- `destroyAllWindows()`:销毁所有打开的窗口。
# 3. OpenCV图像特征提取实践
### 3.1 关键点检测
**关键点检测**是图像特征提取的第一步,它用于识别图像中具有显著性或独特性的点。OpenCV提供了多种关键点检测算法,包括:
- **Harris角点检测器:**检测图像中梯度变化较大的点。
- **SIFT(尺度不变特征变换):**检测图像中具有尺度和旋转不变性的点。
- **SURF(加速稳健特征):**检测图像中具有尺度和旋转不变性的点,比SIFT更快速。
**代码块:**
```python
import cv2
import numpy as np
# 加载图像
image = cv2.imread('image.jpg')
# 使用Harris角点检测器检测关键点
keypoints = cv2.cornerHarris(cv2.cvtColor(image, cv2.COLOR_BGR2GRAY), 2, 3, 0.04)
# 阈值化关键点
keypoints = np.where(keypoints > 0.01 * keypoints.max())
# 绘制关键点
cv2.drawKeypoints(image, keypoints, image)
# 显示图像
cv2.imshow('Keypoints', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
1. `cv2.cornerHarris`函数使用Harris角点检测器检测关键点。
2. `np.where`函数根据阈值筛选关键点。
3. `cv2.drawKeypoints`函数在图像上绘制关键点。
### 3.2 描述符提取
**描述符提取**是图像特征提取的第二步,它用于提取关键点周围图像区域的特征。OpenCV提供了多种描述符提取算法,包括:
- **SIFT描述符:**提取具有尺度和旋转不变性的描述符。
- **SURF描述符:**提取具有尺度和旋转不变性的描述符,比SIFT更快速。
- **ORB(定向快速二进制模式):**提取具有旋转不变性的描述符,比SIFT和SURF更快速。
**代码块:**
```python
import cv2
import numpy as np
# 加载图像
image = cv2.imread('image.jpg')
# 使用SIFT描述符提取器提取描述符
sift = cv2.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(cv2.cvtColor(image, cv2.COLOR_BGR2GRAY), None)
# 打印描述符的形状
print(
```
0
0
相关推荐
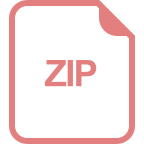
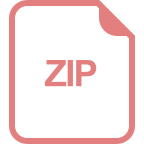
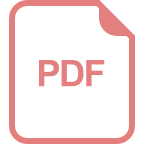
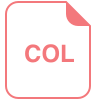
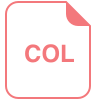
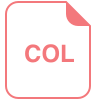
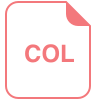
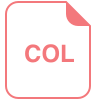
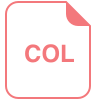