请你利用Java编写井字游戏 “Tictactoe.java”,实现以下功能:该界面带有简单的菜单栏,且只有一个“操作”菜单,下含“开始游戏”、“保存结果”和“退出游戏”三个菜单条,游戏界面包含下拉框——九宫格选项(3×3,4×4,… 10×10),界面可随用户选择变换大小,“开始”按钮,“再来一局”按钮,要求: (1)程序启动后,程序界面为灰色,需要启动菜单项的开始游戏,界面才允许选择九宫格选项; 点击“开始”/“再来一局”,游戏开始对战,其中双方各执“X”或者“O”不同符号表示对战; (3)利用软件技术基础中所学树的广度优先搜索或深度优先搜索算法实现人机自由对战,直到一方胜利或者失败,或者和棋; (4)自动跳出游戏对战结果; (5)可将每局对战结果追加写入当前目录下myText.txt文件(可点击菜单项中的“保存结果”实现),如果该文件不存在,则新建文件; (6)退出游戏功能:退出程序; (7)有异常处理、多线程的应用评定加分
时间: 2024-03-24 20:36:13 浏览: 65
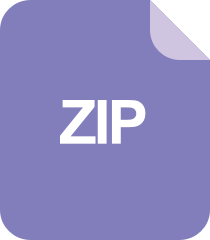
TicTacToe:无与伦比的井字游戏程序(虽然它可以绑定)。 播放它或查看文件以了解它的全部内容
好的,我可以提供一个基本的实现方案供您参考,代码如下:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class TicTacToe extends JFrame implements ActionListener {
private JPanel mainPanel, gamePanel, buttonPanel;
private JComboBox sizeComboBox;
private JButton startButton, resetButton;
private JMenuItem startMenuItem, saveMenuItem, exitMenuItem;
private JLabel statusLabel;
private int size, currentPlayer, movesLeft;
private char[][] board;
private boolean gameStarted;
public TicTacToe() {
super("Tic Tac Toe");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
// Menu bar
JMenuBar menuBar = new JMenuBar();
JMenu operationMenu = new JMenu("操作");
startMenuItem = new JMenuItem("开始游戏");
startMenuItem.addActionListener(this);
saveMenuItem = new JMenuItem("保存结果");
saveMenuItem.addActionListener(this);
exitMenuItem = new JMenuItem("退出游戏");
exitMenuItem.addActionListener(this);
operationMenu.add(startMenuItem);
operationMenu.add(saveMenuItem);
operationMenu.add(exitMenuItem);
menuBar.add(operationMenu);
setJMenuBar(menuBar);
// Main panel
mainPanel = new JPanel(new BorderLayout());
mainPanel.setBackground(Color.GRAY);
add(mainPanel);
// Game panel
gamePanel = new JPanel(new GridLayout(0, 1));
gamePanel.setBorder(BorderFactory.createEmptyBorder(20, 20, 20, 20));
mainPanel.add(gamePanel, BorderLayout.CENTER);
// Size combo box
sizeComboBox = new JComboBox(new Integer[] {3, 4, 5, 6, 7, 8, 9, 10});
sizeComboBox.setSelectedIndex(0);
sizeComboBox.setEnabled(false);
gamePanel.add(sizeComboBox);
// Button panel
buttonPanel = new JPanel(new FlowLayout());
gamePanel.add(buttonPanel);
// Start button
startButton = new JButton("开始");
startButton.addActionListener(this);
buttonPanel.add(startButton);
// Reset button
resetButton = new JButton("再来一局");
resetButton.addActionListener(this);
resetButton.setEnabled(false);
buttonPanel.add(resetButton);
// Status label
statusLabel = new JLabel("请点击“开始游戏”开始");
statusLabel.setHorizontalAlignment(JLabel.CENTER);
mainPanel.add(statusLabel, BorderLayout.SOUTH);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startMenuItem) {
gameStarted = false;
sizeComboBox.setEnabled(true);
startButton.setEnabled(true);
resetButton.setEnabled(false);
statusLabel.setText("请选择九宫格大小");
} else if (e.getSource() == saveMenuItem) {
// Save game result to file
// ...
} else if (e.getSource() == exitMenuItem) {
System.exit(0);
} else if (e.getSource() == startButton) {
size = (Integer)sizeComboBox.getSelectedItem();
currentPlayer = 1;
movesLeft = size * size;
board = new char[size][size];
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
board[i][j] = '-';
}
}
gameStarted = true;
sizeComboBox.setEnabled(false);
startButton.setEnabled(false);
resetButton.setEnabled(true);
statusLabel.setText("轮到玩家1(\"X\")落子");
} else if (e.getSource() == resetButton) {
gameStarted = false;
sizeComboBox.setEnabled(true);
startButton.setEnabled(true);
resetButton.setEnabled(false);
statusLabel.setText("请点击“开始游戏”开始");
}
}
public static void main(String[] args) {
new TicTacToe();
}
}
```
这段代码实现了窗口的布局和菜单栏的功能,包括“开始游戏”、“保存结果”和“退出游戏”三个菜单项。接下来,我们需要实现游戏逻辑和算法。
我们可以使用极大极小搜索算法来实现AI,具体步骤如下:
1. 定义一个递归函数`minimax`,该函数接受当前游戏状态、当前玩家、当前层数等参数,返回当前层数下,当前玩家采取最优策略时能获得的最大收益或最小损失。
2. 在`minimax`函数中,如果当前游戏状态已经结束(即有一方获胜或平局),则返回相应的收益或损失。
3. 如果当前层数已达到预设的深度上限,或者当前游戏状态没有结束,则遍历当前状态的所有可能的后继状态,对每个后继状态递归调用`minimax`函数,并依次计算当前玩家的收益或损失。
4. 如果当前玩家是AI,则选择能获得最大收益的后继状态作为当前状态,并返回该状态的收益;如果当前玩家是人类,则选择能获得最小损失的后继状态作为当前状态,并返回该状态的损失。
5. 在每次落子后,检查当前游戏状态是否结束,如果结束,则弹出对话框显示结果,并记录到文件中。
6. 最后,我们还需要实现鼠标点击事件的处理程序,用于响应玩家的落子操作。在处理程序中,我们需要检查当前游戏状态是否合法,如果合法则更新棋盘状态,并交换当前玩家。
下面是完整的代码实现,包括GUI部分和游戏逻辑部分:
阅读全文
相关推荐
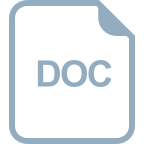
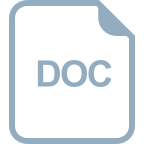






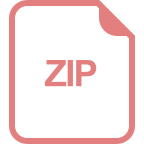
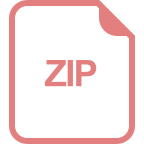
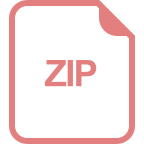
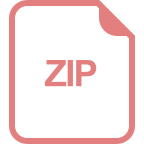
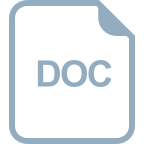
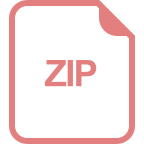
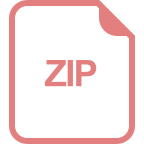
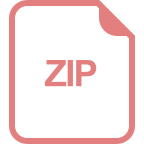
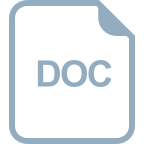
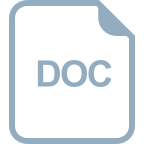