请用vscode编写python代码,利用opencv实现交通灯颜色(红,绿,蓝)的识别功能
时间: 2024-11-23 21:50:46 浏览: 8
在VSCode中编写Python代码以使用OpenCV进行交通灯颜色(红、绿、蓝)识别,首先需要安装必要的库,如`opencv-python`和`numpy`。以下是简单的步骤:
1. **设置环境**:
- 安装VSCode:免费下载并安装Visual Studio Code,它是轻量级的代码编辑器。
- 安装Python和虚拟环境管理工具(如venv或pyenv)。
- 创建一个新的虚拟环境,并激活它。
2. **创建项目文件夹**:
- 在VSCode中新建一个工作区,例如名为"TrafficLightDetector"。
3. **安装所需库**:
- 打开终端(Terminal)或命令提示符(Command Prompt),运行以下命令安装所需的库:
```
pip install opencv-python numpy imutils colorsys
```
4. **编写代码**:
```python
# 导入必要的库
import cv2
import numpy as np
from imutils.video import VideoStream
# 初始化视频流
vs = cv2.VideoCapture(0) # 使用默认摄像头,如果需要文件输入,替换为'video.mp4'
while True:
# 读取帧
ret, frame = vs.read()
if not ret:
break
# 转换到灰度图像便于处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 定义每个交通灯颜色的HSV范围
red_lower = (0, 50, 50)
red_upper = (10, 255, 255)
green_lower = (35, 50, 50)
green_upper = (70, 255, 255)
blue_lower = (100, 50, 50)
blue_upper = (130, 255, 255)
# 检测颜色区域
red_mask = cv2.inRange(gray, red_lower, red_upper)
green_mask = cv2.inRange(gray, green_lower, green_upper)
blue_mask = cv2.inRange(gray, blue_lower, blue_upper)
# 计算每个颜色的面积
_, contours, _ = cv2.findContours(red_mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
red_area = cv2.contourArea(contours[0]) if len(contours) > 0 else 0
_, contours, _ = cv2.findContours(green_mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
green_area = cv2.contourArea(contours[0]) if len(contours) > 0 else 0
_, contours, _ = cv2.findContours(blue_mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
blue_area = cv2.contourArea(contours[0]) if len(contours) > 0 else 0
# 根据面积判断当前颜色
max_color = max(red_area, green_area, blue_area)
if max_color == red_area:
color = "Red"
elif max_color == green_area:
color = "Green"
else:
color = "Blue"
# 显示结果
cv2.putText(frame, color, (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
cv2.imshow("Traffic Light Detection", frame)
key = cv2.waitKey(1)
if key & 0xFF == ord('q'):
break
# 释放资源
vs.release()
cv2.destroyAllWindows()
```
阅读全文
相关推荐
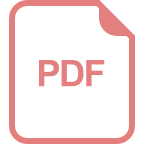
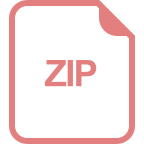
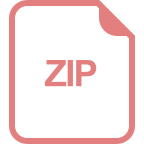





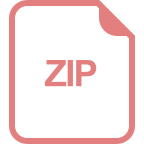
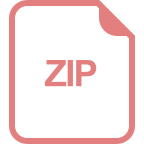
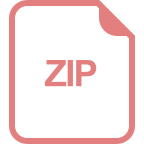
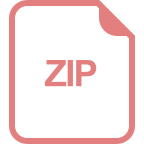
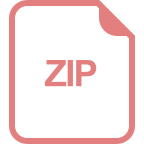
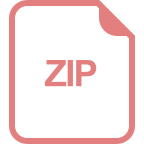
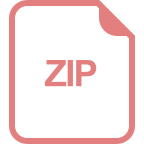