c++编写二维码条码扫码
时间: 2023-07-01 15:25:23 浏览: 198
好的,以下是一个基于 C++ 和 OpenCV 库的二维码/条码扫码示例代码:
```
#include <iostream>
#include <opencv2/opencv.hpp>
#include <opencv2/imgproc.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/dnn.hpp>
using namespace std;
using namespace cv;
using namespace cv::dnn;
int main()
{
// 加载模型和标签
String model_file = "frozen_inference_graph.xml";
String label_file = "label_map.pbtxt";
Net net = readNetFromTensorflow(model_file);
std::vector<String> labels;
std::ifstream ifs(label_file.c_str());
std::string line;
while (std::getline(ifs, line))
{
if (line.find("display_name:") != std::string::npos)
{
labels.push_back(line.substr(15, line.length() - 16));
}
}
// 打开摄像头
VideoCapture cap(0);
if (!cap.isOpened())
{
cout << "Failed to open camera." << endl;
return -1;
}
// 循环读取图像并识别二维码/条码
Mat frame;
while (cap.read(frame))
{
// 转换为灰度图像
Mat gray;
cvtColor(frame, gray, COLOR_BGR2GRAY);
// 识别二维码/条码
std::vector<Mat> codes;
QRCodeDetector detector;
String data = detector.detectAndDecode(gray, codes);
// 显示结果
if (!data.empty())
{
putText(frame, data, Point(50, 50), FONT_HERSHEY_SIMPLEX, 1, Scalar(0, 0, 255), 2);
}
else
{
Mat blob = blobFromImage(frame, 1.0, Size(300, 300), Scalar(127.5, 127.5, 127.5), true, false);
net.setInput(blob);
Mat detections = net.forward();
Mat detectionMat(detections.size[2], detections.size[3], CV_32F, detections.ptr<float>());
for (int i = 0; i < detectionMat.rows; i++)
{
float confidence = detectionMat.at<float>(i, 2);
if (confidence > 0.5)
{
int classId = static_cast<int>(detectionMat.at<float>(i, 1));
String className = labels[classId - 1];
int x1 = static_cast<int>(detectionMat.at<float>(i, 3) * frame.cols);
int y1 = static_cast<int>(detectionMat.at<float>(i, 4) * frame.rows);
int x2 = static_cast<int>(detectionMat.at<float>(i, 5) * frame.cols);
int y2 = static_cast<int>(detectionMat.at<float>(i, 6) * frame.rows);
rectangle(frame, Point(x1, y1), Point(x2, y2), Scalar(0, 0, 255));
putText(frame, className, Point(x1, y1 - 10), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 0, 255));
}
}
}
imshow("Barcode Scanner", frame);
if (waitKey(1) == 27)
{
break;
}
}
// 释放资源
cap.release();
destroyAllWindows();
return 0;
}
```
上述代码首先加载模型文件和标签文件,然后打开摄像头并循环读取图像。对于每帧图像,首先将图像转换为灰度图像,然后使用 OpenCV 的 QRCodeDetector 类识别二维码/条码。如果识别到了二维码/条码,则在图像中绘制识别结果。否则,使用 OpenCV 的 dnn 模块和 TensorFlow 模型识别物体,并在图像中绘制识别结果。
需要注意的是,上述代码仅适用于识别单个二维码/条码,并且对于复杂图像中的二维码/条码识别效果可能不理想。如果需要更高效、更准确的二维码/条码识别算法,可以考虑使用深度学习模型或者其他高级算法。
阅读全文
相关推荐





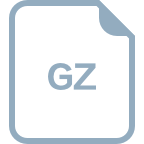












