请给我一个大一能写出的简单的飞机购票系统c语言
时间: 2023-08-04 18:04:39 浏览: 45
好的,以下是一个适合大一学生的简单飞机购票系统C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FLIGHTS 5
#define MAX_NAME 50
typedef struct {
char flight_number[MAX_NAME];
char departure_city[MAX_NAME];
char arrival_city[MAX_NAME];
char departure_time[MAX_NAME];
char arrival_time[MAX_NAME];
int available_seats;
float ticket_price;
} Flight;
Flight flights[MAX_FLIGHTS];
int num_flights = 0;
void add_flight() {
if (num_flights >= MAX_FLIGHTS) {
printf("Sorry, the system can't add more flights!\n");
return;
}
printf("Please enter the flight number: ");
scanf("%s", flights[num_flights].flight_number);
printf("Please enter the departure city: ");
scanf("%s", flights[num_flights].departure_city);
printf("Please enter the arrival city: ");
scanf("%s", flights[num_flights].arrival_city);
printf("Please enter the departure time: ");
scanf("%s", flights[num_flights].departure_time);
printf("Please enter the arrival time: ");
scanf("%s", flights[num_flights].arrival_time);
printf("Please enter the number of available seats: ");
scanf("%d", &flights[num_flights].available_seats);
printf("Please enter the ticket price: ");
scanf("%f", &flights[num_flights].ticket_price);
num_flights++;
printf("The flight has been added successfully!\n");
}
void display_flights() {
printf("Flight Number\tDeparture City\tArrival City\tDeparture Time\tArrival Time\tAvailable Seats\tTicket Price\n");
for (int i = 0; i < num_flights; i++) {
printf("%s\t\t%s\t\t%s\t\t%s\t%s\t%d\t\t%.2f\n", flights[i].flight_number, flights[i].departure_city, flights[i].arrival_city, flights[i].departure_time, flights[i].arrival_time, flights[i].available_seats, flights[i].ticket_price);
}
}
void book_flight() {
char flight_number[MAX_NAME];
int num_seats;
printf("Please enter the flight number you want to book: ");
scanf("%s", flight_number);
for (int i = 0; i < num_flights; i++) {
if (strcmp(flights[i].flight_number, flight_number) == 0) {
printf("Please enter the number of seats you want to book: ");
scanf("%d", &num_seats);
if (num_seats > flights[i].available_seats) {
printf("Sorry, there are not enough available seats on this flight.\n");
return;
}
flights[i].available_seats -= num_seats;
float total_price = num_seats * flights[i].ticket_price;
printf("The booking has been made successfully! The total price is %.2f. Enjoy your flight.\n", total_price);
return;
}
}
printf("Sorry, the flight number you entered is not valid.\n");
}
int main() {
int option;
do {
printf("\n\n");
printf("Welcome to the airline booking system!\n");
printf("1. Add a flight\n");
printf("2. Display all flights\n");
printf("3. Book a flight\n");
printf("4. Exit\n");
printf("Please enter your choice: ");
scanf("%d", &option);
switch (option) {
case 1:
add_flight();
break;
case 2:
display_flights();
break;
case 3:
book_flight();
break;
case 4:
printf("Thank you for using the system. Goodbye!\n");
exit(0);
default:
printf("Sorry, the option you entered is not valid.\n");
}
} while (1);
return 0;
}
```
以上代码实现了一个简单的飞机购票系统,包括添加航班、展示所有航班、预订航班等功能。在这个简单的系统中,每个航班包括起飞城市、到达城市、起飞时间、到达时间、可用座位数和票价等信息。用户可以选择预订航班,并输入所需的座位数,系统会根据座位数计算总价并输出。
相关推荐
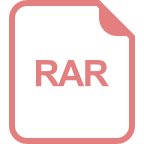













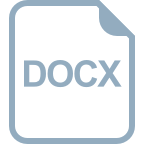