如何对随机森林模型做aic检验,python代码
时间: 2024-09-25 17:07:55 浏览: 40
随机森林模型的AIC (Akaike Information Criterion) 检验主要是用来评估模型复杂度的一个统计量,它结合了模型的拟合优度(通过log似然函数衡量)和模型的复杂度(由自由度表示)。AIC越小,说明模型的预测效果越好,而过度复杂的模型可能会过拟合,导致较差的泛化能力。
在Python中,scikit-learn库的`sklearn.model_selection.GridSearchCV`可以用于交叉验证,并计算每个模型的AIC。下面是一个简单的例子:
```python
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import GridSearchCV
import numpy as np
# 加载数据
iris = load_iris()
X, y = iris.data, iris.target
# 定义参数网格
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 10, 20]
}
# 创建RandomForestClassifier实例
rf = RandomForestClassifier()
# 使用GridSearchCV进行模型训练并获取最优模型和AIC值
grid_search = GridSearchCV(rf, param_grid, cv=5, scoring='neg_mean_squared_error', return_train_score=True)
grid_search.fit(X, y)
# 最优模型
best_rf = grid_search.best_estimator_
print("Best Model: ", best_rf)
# 计算AIC
aic = -2 * grid_search.cv_results_['mean_test_score'] + 2 * len(grid_search.best_params_)
print("AIC: ", aic)
# 查看所有模型的AIC
results = grid_search.cv_results_['mean_test_score']
aicc = np.array(results) - ((len(results) - 1) * np.log(len(results)))
best_aicc_index = np.argmin(aicc)
print(f"Model with the lowest AICc: {best_aicc_index}")
```
在这个例子中,我们首先设置了随机森林的不同参数组合,然后在交叉验证下测试每种设置,最后选取AIC最小的模型作为最佳模型。`GridSearchCV`返回的结果包含了每个模型的性能指标,从中我们可以提取出AIC的值。
阅读全文
相关推荐
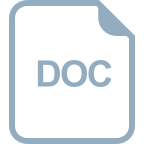
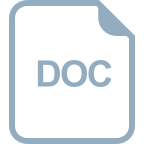
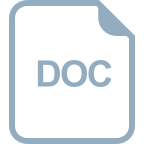

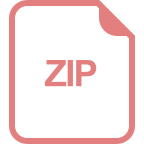
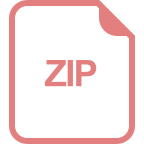
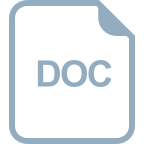
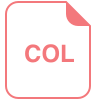
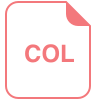
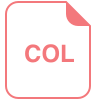
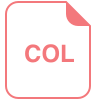
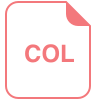
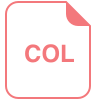
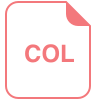
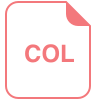
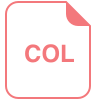
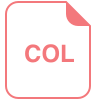
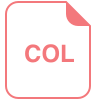
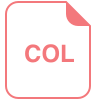