用c语言编制一个能演示执行集合的交、并和差运算的程序。 【任务要求】 1)集合元素用小写英文字母,执行各种操作应以对话方式执行。 2)算法要点:利用单链表表示集合;理解好三种运算的含义
时间: 2024-05-16 11:16:09 浏览: 101
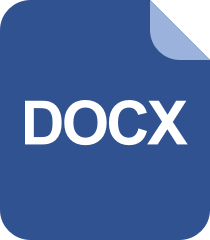
集合的并、交和差运算的算法.docx
好的,下面是一个用C语言编写的集合交、并和差运算的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义集合元素结构体
typedef struct Element {
char value; // 元素值
struct Element *next; // 指向下一个元素的指针
} Element;
// 定义集合结构体
typedef struct Set {
Element *head; // 指向第一个元素的指针
} Set;
// 创建一个空集合
Set *createSet() {
Set *set = (Set *) malloc(sizeof(Set));
set->head = NULL;
return set;
}
// 判断元素是否在集合中
int contains(Set *set, char value) {
Element *current = set->head;
while (current != NULL) {
if (current->value == value) {
return 1; // 找到了
}
current = current->next;
}
return 0; // 没找到
}
// 添加元素到集合中
void add(Set *set, char value) {
// 如果元素已经存在,则不添加
if (contains(set, value)) {
return;
}
// 创建一个新元素
Element *newElement = (Element *) malloc(sizeof(Element));
newElement->value = value;
newElement->next = NULL;
// 如果集合为空,则直接将新元素设置为头元素
if (set->head == NULL) {
set->head = newElement;
return;
}
// 找到集合中的最后一个元素
Element *current = set->head;
while (current->next != NULL) {
current = current->next;
}
// 将新元素添加到最后一个元素的后面
current->next = newElement;
}
// 从集合中删除元素
void removeElement(Set *set, char value) {
// 如果集合为空,则直接返回
if (set->head == NULL) {
return;
}
// 如果要删除的是头元素,则直接将头指针指向下一个元素
if (set->head->value == value) {
Element *temp = set->head;
set->head = set->head->next;
free(temp);
return;
}
// 找到要删除元素的前一个元素
Element *prev = set->head;
while (prev->next != NULL && prev->next->value != value) {
prev = prev->next;
}
// 如果找到了要删除的元素,则删除
if (prev->next != NULL) {
Element *temp = prev->next;
prev->next = temp->next;
free(temp);
}
}
// 打印集合中的元素
void printSet(Set *set) {
printf("{ ");
Element *current = set->head;
while (current != NULL) {
printf("%c ", current->value);
current = current->next;
}
printf("}\n");
}
int main() {
Set *set1 = createSet();
Set *set2 = createSet();
Set *set3 = createSet();
char input[100];
char command[10];
char value;
while (1) {
printf("请输入命令:");
fgets(input, sizeof(input), stdin);
sscanf(input, "%s %c", command, &value);
if (strcmp(command, "add1") == 0) {
add(set1, value);
printf("集合1中添加元素%c后,当前集合为:", value);
printSet(set1);
} else if (strcmp(command, "add2") == 0) {
add(set2, value);
printf("集合2中添加元素%c后,当前集合为:", value);
printSet(set2);
} else if (strcmp(command, "remove1") == 0) {
removeElement(set1, value);
printf("从集合1中删除元素%c后,当前集合为:", value);
printSet(set1);
} else if (strcmp(command, "remove2") == 0) {
removeElement(set2, value);
printf("从集合2中删除元素%c后,当前集合为:", value);
printSet(set2);
} else if (strcmp(command, "intersect") == 0) {
Element *current = set1->head;
while (current != NULL) {
if (contains(set2, current->value)) {
add(set3, current->value);
}
current = current->next;
}
printf("集合1与集合2的交集为:");
printSet(set3);
set3 = createSet(); // 清空集合3
} else if (strcmp(command, "union") == 0) {
Element *current = set1->head;
while (current != NULL) {
add(set3, current->value);
current = current->next;
}
current = set2->head;
while (current != NULL) {
add(set3, current->value);
current = current->next;
}
printf("集合1与集合2的并集为:");
printSet(set3);
set3 = createSet(); // 清空集合3
} else if (strcmp(command, "difference") == 0) {
Element *current = set1->head;
while (current != NULL) {
if (!contains(set2, current->value)) {
add(set3, current->value);
}
current = current->next;
}
printf("集合1与集合2的差集为:");
printSet(set3);
set3 = createSet(); // 清空集合3
} else if (strcmp(command, "exit") == 0) {
break;
} else {
printf("无效的命令!\n");
}
}
return 0;
}
```
运行程序后,可以通过输入不同的命令来执行集合的交、并和差运算。例如:
```
请输入命令:add1 a
集合1中添加元素a后,当前集合为:{ a }
请输入命令:add1 b
集合1中添加元素b后,当前集合为:{ a b }
请输入命令:add1 c
集合1中添加元素c后,当前集合为:{ a b c }
请输入命令:add2 b
集合2中添加元素b后,当前集合为:{ b }
请输入命令:add2 d
集合2中添加元素d后,当前集合为:{ b d }
请输入命令:intersect
集合1与集合2的交集为:{ b }
请输入命令:union
集合1与集合2的并集为:{ a b c d }
请输入命令:difference
集合1与集合2的差集为:{ a c }
请输入命令:remove1 c
从集合1中删除元素c后,当前集合为:{ a b }
请输入命令:exit
```
阅读全文
相关推荐
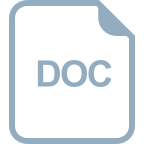











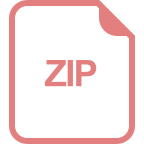