编写决策树算法实现心脏病预测
时间: 2024-05-23 13:13:45 浏览: 102
好的,下面我给您介绍一下使用决策树算法实现心脏病预测的步骤:
1. 数据收集:收集具有代表性的心脏病患者的医疗数据集。
2. 数据预处理:对数据进行清洗、特征选择和特征提取等预处理操作,使得数据能够被决策树算法处理。
3. 划分训练集和测试集:将数据集按照一定比例划分为训练集和测试集,一般使用 70% 的数据作为训练集,30% 的数据作为测试集。
4. 训练模型:使用训练集训练决策树模型,一般采用 ID3、C4.5、CART 等算法进行训练。
5. 模型评估:使用测试集对训练好的模型进行评估,一般使用准确率、召回率、F1 值等指标进行评价。
6. 模型优化:根据评估结果,对模型进行优化,如调整模型参数、增加特征等。
7. 预测结果:使用优化后的模型对新的心脏病患者进行预测,得到预测结果。
以上是使用决策树算法实现心脏病预测的基本步骤。具体实现时,您可以使用 Python 中的 scikit-learn 库来实现。
相关问题
自己编写决策树算法实现心脏病预测
首先,需要收集一个心脏病的数据集,并将其分为训练集和测试集。然后,可以按照以下步骤实现决策树算法:
1. 计算每个特征的信息增益,以选择最佳的特征进行节点拆分。
2. 以信息增益最高的特征作为根节点进行拆分,并生成两个子节点。
3. 对于每个子节点,重复步骤1和2,直到达到预定义的停止条件(如达到最大深度或没有更多数据可拆分)。
4. 在终端节点处将数据分配给最常见的类别。
下面是一个简单的 Python 代码实现:
```
import numpy as np
class DecisionTree:
def __init__(self, max_depth=5):
self.max_depth = max_depth
def fit(self, X, y):
self.tree = self.build_tree(X, y, 0)
def predict(self, X):
return np.array([self.traverse(x, self.tree) for x in X])
def build_tree(self, X, y, depth):
n_samples, n_features = X.shape
n_labels = len(np.unique(y))
# stop if maximum depth reached or only one class present
if depth == self.max_depth or n_labels == 1:
return np.argmax(np.bincount(y))
# calculate information gain for each feature
best_feature, best_gain = None, -1
for i in range(n_features):
gain = self.information_gain(X[:, i], y)
if gain > best_gain:
best_feature = i
best_gain = gain
# stop if no information gain made
if best_gain == 0:
return np.argmax(np.bincount(y))
# split data using best feature
left_mask = X[:, best_feature] <= np.median(X[:, best_feature])
right_mask = X[:, best_feature] > np.median(X[:, best_feature])
left_tree = self.build_tree(X[left_mask], y[left_mask], depth+1)
right_tree = self.build_tree(X[right_mask], y[right_mask], depth+1)
# create node dictionary
node = {
'feature': best_feature,
'median': np.median(X[:, best_feature]),
'left': left_tree,
'right': right_tree
}
return node
def information_gain(self, feature, y):
n_samples = len(y)
entropy_parent = self.entropy(y)
# split data based on median of feature
left_mask = feature <= np.median(feature)
right_mask = feature > np.median(feature)
n_left, n_right = len(y[left_mask]), len(y[right_mask])
# calculate entropy of children nodes
entropy_left = self.entropy(y[left_mask])
entropy_right = self.entropy(y[right_mask])
# calculate weighted average of entropy for children nodes
entropy_children = (n_left/n_samples)*entropy_left + (n_right/n_samples)*entropy_right
# calculate information gain
information_gain = entropy_parent - entropy_children
return information_gain
def entropy(self, y):
n_samples = len(y)
_, counts = np.unique(y, return_counts=True)
probabilities = counts / n_samples
entropy = -np.sum(probabilities * np.log2(probabilities))
return entropy
def traverse(self, x, node):
if isinstance(node, int):
return node
elif x[node['feature']] <= node['median']:
return self.traverse(x, node['left'])
else:
return self.traverse(x, node['right'])
```
这个决策树实现使用信息增益作为节点拆分的标准,停止条件为达到最大深度或只有一个类别。它还包括一个entropy()函数,用于计算数据集的熵,以便计算信息增益。在build_tree()函数中,它首先计算每个特征的信息增益,选择具有最高增益的特征进行拆分,并递归地构建决策树。在predict()函数中,它使用递归遍历决策树来预测每个样本的类别。
用python编写决策树算法实现心脏病预测
好的,下面是用Python编写的决策树算法实现心脏病预测的示例代码:
```python
# 导入必要的库
import pandas as pd
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 读取数据集
data = pd.read_csv('heart.csv')
# 划分特征和标签
X = data.iloc[:, :-1]
y = data.iloc[:, -1]
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义决策树模型
dtc = DecisionTreeClassifier()
# 训练模型
dtc.fit(X_train, y_train)
# 预测测试集
y_pred = dtc.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print('决策树模型的准确率为:', accuracy)
```
在此代码中,我们使用了Pandas库来读取我们的数据集,并使用sklearn库中的`DecisionTreeClassifier`类来定义我们的决策树模型。我们还使用了`train_test_split`函数来划分训练集和测试集,并使用`accuracy_score`函数来计算我们模型的准确率。最后,我们输出了模型的准确率。
需要注意的是,这里的数据集是来自Kaggle平台上的一个心脏病数据集,你可以在Kaggle上搜索"heart disease UCI"来下载该数据集。
阅读全文
相关推荐
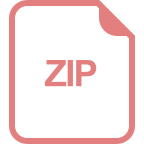
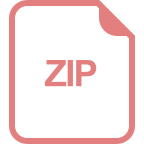









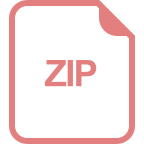
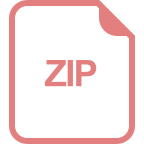
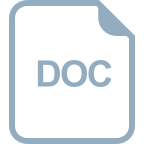
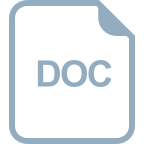
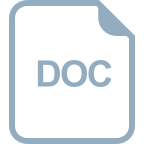