oauth2requestfactory
时间: 2023-12-08 07:02:05 浏览: 29
OAuth2RequestFactory是一个用于创建OAuth2请求的工厂类。在使用OAuth2进行身份验证和授权时,我们需要发送各种类型的请求来与认证服务器进行交互。OAuth2RequestFactory的作用就是简化了请求的创建过程,提供了一种方便的方式来构建OAuth2请求。
使用OAuth2RequestFactory,我们可以根据需要创建不同类型的请求对象,如获取授权码请求、获取访问令牌请求等。通过指定请求的参数和细节,我们可以定制化地构建每个请求。这包括指定请求的URL、请求方法、请求头部信息等。
OAuth2RequestFactory的另一个重要功能是处理身份验证信息和令牌的存储和传递。在OAuth2过程中,通常需要将身份验证信息和令牌添加到请求中,以便进行身份验证和授权。OAuth2RequestFactory可以从存储中检索这些信息,并将其添加到相应的请求中,省去了手动处理这些细节的过程。
总而言之,OAuth2RequestFactory是一个方便的工具类,用于简化OAuth2请求的创建和处理过程。通过使用OAuth2RequestFactory,我们可以更加轻松地构建OAuth2请求,并且可以灵活地处理身份验证和授权所需的信息。这大大简化了使用OAuth2进行身份验证和授权的开发工作。
相关问题
springboot集成oauth2
Spring Boot 集成 OAuth2 可以实现授权和认证功能,可以为我们的应用程序提供更加安全的访问控制。
下面是实现 Spring Boot 集成 OAuth2 的步骤:
1. 添加依赖
```
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.1.RELEASE</version>
</dependency>
```
2. 配置 Spring Security
在 `WebSecurityConfigurerAdapter` 类中配置 OAuth2 安全配置。
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private ClientDetailsService clientDetailsService;
@Autowired
public void globalUserDetails(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user1").password("password1").roles("USER")
.and()
.withUser("admin1").password("password2").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/oauth/token").permitAll()
.antMatchers("/api/**").authenticated()
.and().csrf().disable()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService());
}
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Bean
public TokenStore tokenStore() {
return new InMemoryTokenStore();
}
@Bean
@Autowired
public ApprovalStore approvalStore(TokenStore tokenStore) throws Exception {
TokenApprovalStore store = new TokenApprovalStore();
store.setTokenStore(tokenStore);
return store;
}
@Bean
@Autowired
public AuthorizationCodeServices authorizationCodeServices(TokenStore tokenStore) {
return new JdbcAuthorizationCodeServices(dataSource());
}
@Bean
@Autowired
public OAuth2RequestFactory requestFactory(ClientDetailsService clientDetailsService) {
return new DefaultOAuth2RequestFactory(clientDetailsService);
}
@Bean
@Autowired
public OAuth2AuthenticationEntryPoint authenticationEntryPoint(ClientDetailsService clientDetailsService) {
OAuth2AuthenticationEntryPoint entryPoint = new OAuth2AuthenticationEntryPoint();
entryPoint.setRealmName("oauth/client");
entryPoint.setClientDetailsService(clientDetailsService);
return entryPoint;
}
@Bean
@Autowired
public OAuth2AccessDeniedHandler accessDeniedHandler() {
return new OAuth2AccessDeniedHandler();
}
@Autowired
private DataSource dataSource;
@Bean
public JdbcTokenStore tokenStore(DataSource dataSource) {
return new JdbcTokenStore(dataSource);
}
@Bean
public JdbcClientDetailsService clientDetailsService(DataSource dataSource) {
return new JdbcClientDetailsService(dataSource);
}
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.jdbc(dataSource);
}
}
```
3. 配置 OAuth2
在 `AuthorizationServerConfigurerAdapter` 类中配置 OAuth2 安全配置。
```
@Configuration
@EnableAuthorizationServer
public class OAuth2AuthorizationConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
private TokenStore tokenStore;
@Autowired
private UserApprovalHandler userApprovalHandler;
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private ClientDetailsService clientDetailsService;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("clientapp")
.secret("123456")
.authorizedGrantTypes("password", "refresh_token")
.authorities("USER")
.scopes("read", "write")
.resourceIds("oauth2-resource")
.accessTokenValiditySeconds(600)
.refreshTokenValiditySeconds(6000);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore)
.userApprovalHandler(userApprovalHandler)
.authenticationManager(authenticationManager);
}
@Override
public void configure(AuthorizationServerSecurityConfigurer oauthServer) throws Exception {
oauthServer.realm("oauth/client");
}
@Bean
public TokenStore tokenStore() {
return new InMemoryTokenStore();
}
@Bean
@Autowired
public ApprovalStore approvalStore(TokenStore tokenStore) throws Exception {
TokenApprovalStore store = new TokenApprovalStore();
store.setTokenStore(tokenStore);
return store;
}
@Bean
@Autowired
public OAuth2RequestFactory requestFactory(ClientDetailsService clientDetailsService) {
return new DefaultOAuth2RequestFactory(clientDetailsService);
}
}
```
4. 配置资源服务器
在 `ResourceServerConfigurerAdapter` 类中配置资源服务器。
```
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated();
}
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources.resourceId("oauth2-resource");
}
}
```
5. 测试
启动应用程序并访问 `http://localhost:8080/oauth/token`,使用 `clientapp` 和 `123456` 进行身份验证。如果身份验证成功,应该会收到一个访问令牌。
然后,访问 `http://localhost:8080/api/hello`,应该会看到一个受保护的资源的欢迎消息。
springmvc oauth2
Spring MVC是一个基于Java的Web框架,用于构建Web应用程序。它提供了一种模型-控制器(MVC)的架构模式,使开发人员能够将应用程序的不同方面进行分离,从而实现更好的可维护性和可扩展性。
OAuth2是一种授权框架,用于保护Web应用程序的资源。它允许用户授权第三方应用程序访问其受保护的资源,而无需共享其凭据(如用户名和密码)。OAuth2定义了一组角色(如资源所有者、客户端、授权服务器和资源服务器)以及一组授权流程(如授权码流程、隐式流程和密码流程),用于实现安全的授权机制。
Spring MVC和OAuth2可以结合使用,以实现安全的Web应用程序。Spring Security是Spring框架提供的安全性解决方案,它集成了OAuth2,并提供了一些便捷的注解和配置选项,用于保护Spring MVC应用程序的资源。
通过使用Spring Security OAuth2,您可以轻松地将OAuth2授权添加到Spring MVC应用程序中。您可以配置客户端详细信息、授权服务器详细信息和资源服务器详细信息,并使用注解来保护您的控制器和方法。此外,Spring Security OAuth2还提供了一些默认的端点和过滤器,用于处理OAuth2授权流程。
总结起来,Spring MVC是一个用于构建Web应用程序的框架,而OAuth2是一种用于保护Web应用程序资源的授权框架。通过结合使用Spring Security OAuth2,您可以轻松地将OAuth2授权添加到Spring MVC应用程序中。
相关推荐
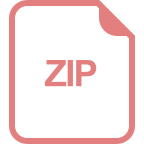












