循环队列 数组c++
时间: 2023-11-15 19:07:20 浏览: 112
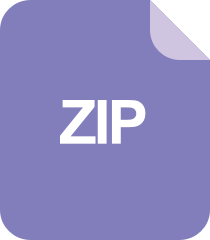
循环队列(C++)
循环队列是一种特殊的队列,它可以充分利用数组空间,实现队列的基本操作。在循环队列中,队列的头尾相连,当队列满时,可以将队列头指针指向数组的第一个位置,实现循环利用。C++中实现循环队列可以使用数组和指针结构体来实现。
以下是使用数组实现循环队列的C++代码:
```c++
#define MAXSIZE 10 // 定义循环队列的最大长度
typedef int ElementType; // 定义队列元素类型
class CircularQueue {
private:
ElementType data[MAXSIZE]; // 队列数组
int front; // 队头指针
int rear; // 队尾指针
public:
CircularQueue() { // 构造函数,初始化队头和队尾指针
front = rear = 0;
}
bool isEmpty() { // 判断队列是否为空
return front == rear;
}
bool isFull() { // 判断队列是否为满
return (rear + 1) % MAXSIZE == front; }
void enQueue(ElementType x) { // 入队
if (isFull()) {
cout << "Queue is full!" << endl;
return;
}
data[rear] = x;
rear = (rear + 1) % MAXSIZE;
}
void deQueue() { // 出队
if (isEmpty()) {
cout << "Queue is empty!" << endl;
return;
}
front = (front + 1) % MAXSIZE;
}
void printQueue() { // 打印队列
if (isEmpty()) {
cout << "Queue is empty!" << endl;
return;
}
int i = front;
while (i != rear) {
cout << data[i] << " ";
i = (i + 1) % MAXSIZE;
}
cout << endl;
}
};
```
使用指针结构体实现循环队列的C++代码如下:
```c++
#define MAXSIZE 10 // 定义循环队列的最大长度
typedef int ElementType; // 定义队列元素类型
struct Queue {
ElementType* data; // 队列数组
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列长度
int capacity; // 队列容量
};
Queue* createQueue(int k) { // 创建空队列
Queue* q = new Queue;
q->data = new ElementType[k];
q->front = q->rear = 0;
q->size = 0;
q->capacity = k;
return q;
}
bool isEmpty(Queue* q) { // 判断队列是否为空
return q->size == 0;
}
bool isFull(Queue* q) { // 判断队列是否为满
return q->size == q->capacity;
}
void makeEmpty(Queue* q) { // 置空
q->front = q->rear = 0;
q->size = 0;
}
int lengthOfQueue(Queue* q) { // 计算队列长度
return q->size;
}
void enQueue(Queue* q, ElementType x) { // 入队
if (isFull(q)) {
cout << "Queue is full!" << endl;
return;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % q->capacity;
q->size++;
}
void deQueue(Queue* q) { // 出队
if (isEmpty(q)) {
cout << "Queue is empty!" << endl;
return;
}
q->front = (q->front + 1) % q->capacity;
q->size--;
}
void printQueue(Queue* q) { // 打印队列
if (isEmpty(q)) {
cout << "Queue is empty!" << endl;
return;
}
int i = q->front;
while (i != q->rear) {
cout << q->data[i] << " ";
i = (i + 1) % q->capacity;
}
cout << endl;
}
```
阅读全文
相关推荐
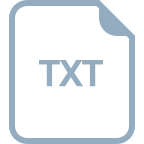
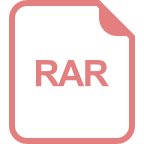
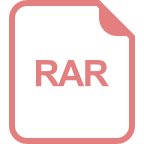
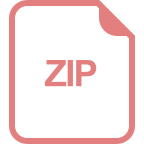
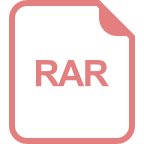
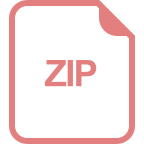
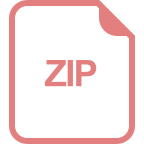
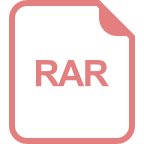
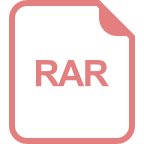
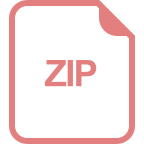
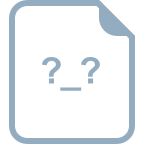
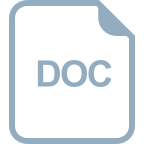
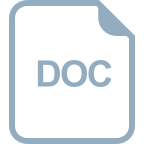
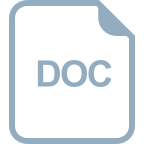
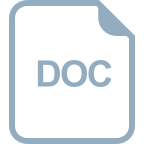
