public class MaxWord { void getMaxWord(String sentence){ String[] words = sentence.split(""); String[] dictionary = new String[words.length]; int[] wordcount = new int[words.length]; Arrays.fill(dictionary,""); Arrays.fill(wordcount,0); for (int i = 0; i < words.length; i++) { String word = words[i]; int wordindex = findWord(xxx); if (wordindex>=0){ wordcount[wordindex]++;; } else { dictionary[dichead]=word; wordcount[dichead]=1; dichead++; } } int maxindex = getMaxIndex(wordcount); System.out.println("Max Count Word:"+dictionary[maxindex]); System.out.println("Max Count:"+wordcount[maxindex]); }}这段代码里的xxx应该写什么?
时间: 2024-02-14 10:14:44 浏览: 25
在 `xxx` 的位置应该写 `word`,因为这里需要查找 `dictionary` 数组中是否存在当前单词。所以完整的代码如下:
```java
public class MaxWord {
void getMaxWord(String sentence){
String[] words = sentence.split(" ");
String[] dictionary = new String[words.length];
int[] wordcount = new int[words.length];
Arrays.fill(dictionary,"");
Arrays.fill(wordcount,0);
int dichead = 0;
for (int i = 0; i < words.length; i++) {
String word = words[i];
int wordindex = findWord(dictionary, word);
if (wordindex>=0){
wordcount[wordindex]++;
}
else {
dictionary[dichead]=word;
wordcount[dichead]=1;
dichead++;
}
}
int maxindex = getMaxIndex(wordcount);
System.out.println("Max Count Word:"+dictionary[maxindex]);
System.out.println("Max Count:"+wordcount[maxindex]);
}
int findWord(String[] dictionary, String word) {
for (int i = 0; i < dictionary.length; i++) {
if (word.equals(dictionary[i])) {
return i;
}
}
return -1;
}
int getMaxIndex(int[] arr) {
int maxindex = 0;
for (int i = 1; i < arr.length; i++) {
if (arr[i]>arr[maxindex]) {
maxindex = i;
}
}
return maxindex;
}
}
```
在这里,我做出了一些改动:
1. `String[] words = sentence.split(" ");`,原来的代码使用了 `split("")`,这会把每个字符都当成一个单词,应该改为 `split(" ")`,使用空格分割单词。
2. 添加了 `findWord` 方法和 `getMaxIndex` 方法,分别用于查找单词在 `dictionary` 数组中的索引和获取 `wordcount` 数组中最大值的索引。
3. 在 `findWord` 方法中,使用 `word.equals(dictionary[i])` 来比较两个字符串是否相等,因为字符串是对象,使用 `==` 只会比较两个字符串对象的引用是否相等,而不是值是否相等。
相关推荐
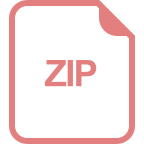
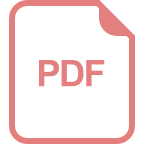
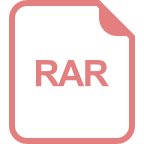







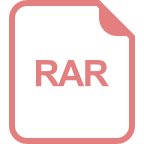
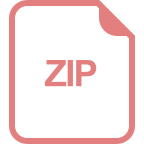
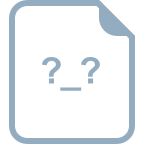
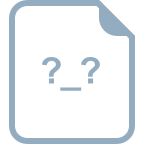
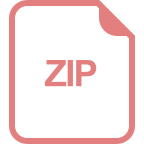