Word 删除 只有数字的行如何在C++中设计一个程序,该程序能够接受用户输入多行文本,统计并返回每行中出现次数最多的关键词(不论重复多少次),并确保程序逻辑清晰且有效?++
时间: 2024-10-18 10:19:48 浏览: 22
在C++中设计这样一个程序,可以分为以下几个步骤:
1. **数据结构选择**:
使用`std::map`来存储每个单词及其出现的次数。键是单词,值是计数。
2. **输入处理**:
创建一个循环来读取用户一行行输入,每次读取后清理字符串,只保留数字字符。你可以使用`std::isdigit()`函数来进行这个操作。
```cpp
#include <iostream>
#include <string>
#include <cctype>
#include <map>
std::string cleanLine(const std::string &line) {
std::string cleaned;
for (char c : line) {
if (std::isdigit(c)) {
cleaned += c;
}
}
return cleaned;
}
```
3. **分析行并更新统计**:
清理后的行将用于更新词频统计。遍历清洗后的字符串,将其分割成单词,并检查是否已经在地图中,如果存在则增加计数,否则插入新的元素。
4. **找出最常见的词**:
当所有输入都处理完毕后,遍历`std::map`以找到出现次数最多的词。这可能需要两个临时变量,一个记录当前最多次数,另一个记录对应的词。
5. **输出结果**:
输出最常见的词以及其出现的次数。
完整的示例代码可能会像这样:
```cpp
int main() {
std::map<std::string, int> wordCount;
std::string line;
while (std::getline(std::cin, line)) {
std::string cleaned = cleanLine(line);
size_t pos = 0;
while ((pos = cleaned.find_first_of(" ")) != std::string::npos) {
std::string word = cleaned.substr(0, pos);
++wordCount[word];
cleaned.erase(0, pos + 1);
}
if (!cleaned.empty()) {
++wordCount[cleaned]; // 处理最后一个单词
}
}
auto maxWord = std::max_element(wordCount.begin(), wordCount.end(),
[](const auto &a, const auto &b) { return a.second < b.second; });
std::cout << "最常见的词是 \"" << maxWord->first << "\", 出现了 " << maxWord->second << " 次。\n";
return 0;
}
```
阅读全文
相关推荐
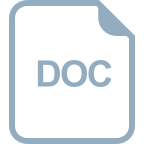
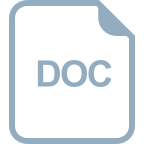
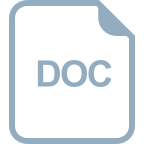

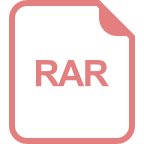
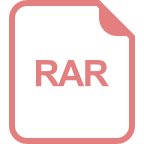
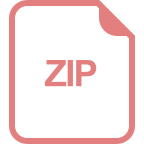
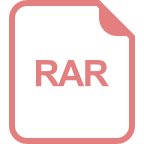
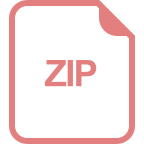
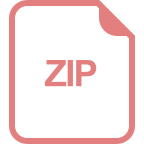
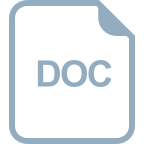
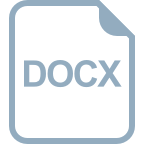
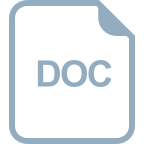
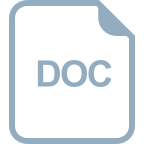
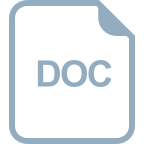
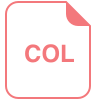


