vue点击按钮的时候,动态生成一个el-input-number 计数器
时间: 2023-11-08 16:19:17 浏览: 127
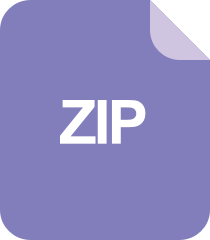
手动动态改变el-tabs标签页顺序
要实现点击按钮动态生成 `el-input-number` 计数器组件,可以使用 `v-if` 条件渲染和 `v-for` 循环渲染的方式,具体如下:
```html
<template>
<div>
<el-button @click="addCounter">添加计数器</el-button>
<div v-for="(counter, index) in counterList" :key="index">
<el-input-number v-model="counter.value" :min="counter.min" :max="counter.max" :step="counter.step"></el-input-number>
</div>
</div>
</template>
<script>
export default {
data() {
return {
counterList: []
}
},
methods: {
addCounter() {
const newCounter = {
value: 0, // 初始值
min: 0, // 最小值
max: 10, // 最大值
step: 1 // 步长
}
this.counterList.push(newCounter)
}
}
}
</script>
```
上面的代码中,我们声明了一个 `counterList` 数组,用于存储所有的计数器对象。在模板中,我们使用 `v-for` 循环渲染 `counterList` 数组中的每一个计数器对象,并使用 `v-model` 把计数器的值绑定到对应的对象上。当点击添加计数器的按钮时,我们向 `counterList` 数组中添加一个新的计数器对象,然后在模板中就能够动态生成一个新的 `el-input-number` 计数器组件了。
当然,你也可以在添加计数器时,通过传递参数的方式来设置计数器的初始化值、最小值、最大值和步长等属性。例如:
```html
<template>
<div>
<el-button @click="addCounter(0, 0, 10, 1)">添加计数器</el-button>
<div v-for="(counter, index) in counterList" :key="index">
<el-input-number v-model="counter.value" :min="counter.min" :max="counter.max" :step="counter.step"></el-input-number>
</div>
</div>
</template>
<script>
export default {
data() {
return {
counterList: []
}
},
methods: {
addCounter(value, min, max, step) {
const newCounter = {
value: value,
min: min,
max: max,
step: step
}
this.counterList.push(newCounter)
}
}
}
</script>
```
这样,当你调用 `addCounter` 方法时,就能够根据传递的参数来动态生成一个带有指定初始值、最小值、最大值和步长的 `el-input-number` 计数器组件了。
阅读全文
相关推荐
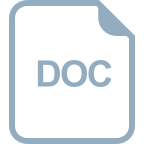
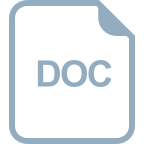



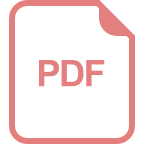
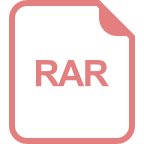
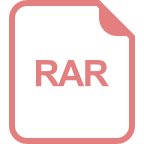
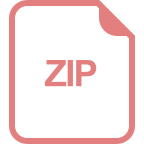
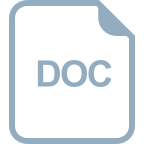
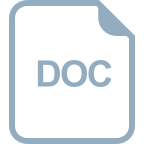
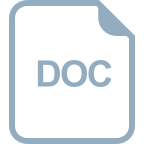
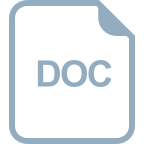
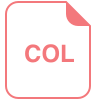


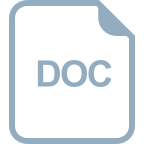
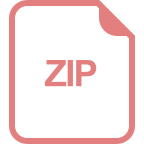