convnext作为主干网络的代码
时间: 2023-09-10 20:11:25 浏览: 100
ConvNets are the backbone of many computer vision models, including image classification, object detection, and segmentation. Here is an example of ConvNet architecture in PyTorch:
```python
import torch.nn as nn
class ConvNet(nn.Module):
def __init__(self):
super(ConvNet, self).__init__()
# Convolutional layers
self.conv1 = nn.Conv2d(in_channels=3, out_channels=32, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(in_channels=32, out_channels=64, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(in_channels=64, out_channels=128, kernel_size=3, stride=1, padding=1)
# Pooling layer
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
# Fully connected layers
self.fc1 = nn.Linear(in_features=128 * 4 * 4, out_features=512)
self.fc2 = nn.Linear(in_features=512, out_features=10)
# Activation function
self.relu = nn.ReLU()
def forward(self, x):
# Convolutional layers
x = self.conv1(x)
x = self.relu(x)
x = self.pool(x)
x = self.conv2(x)
x = self.relu(x)
x = self.pool(x)
x = self.conv3(x)
x = self.relu(x)
x = self.pool(x)
# Flatten the output
x = x.view(-1, 128 * 4 * 4)
# Fully connected layers
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
return x
```
This ConvNet consists of three convolutional layers with ReLU activation and max pooling, followed by two fully connected layers. The input to the network is a 3-channel image of size 32x32, and the output is a tensor of size 10, representing the predicted class probabilities.
阅读全文
相关推荐



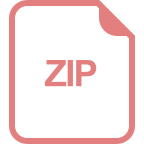
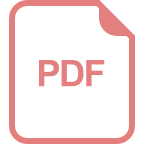
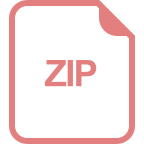
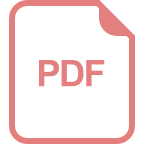
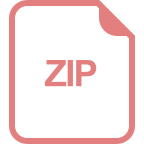
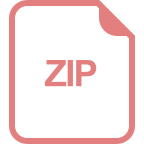
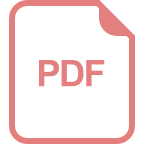
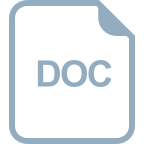
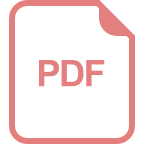
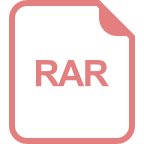
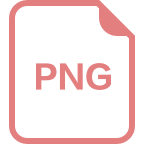