JAVA 使用抽象方法和抽象类,编程实现图5.3所示的类层次图,并给出一个运行结果。
时间: 2024-02-19 12:57:19 浏览: 61
由于无法插入图片,以下是图5.3的类层次结构代码描述:
```
abstract class Shape {
protected String color;
public Shape(String color) {
this.color = color;
}
public abstract double getArea();
@Override
public String toString() {
return "颜色为" + color + "的";
}
}
class Circle extends Shape {
protected double radius;
public Circle(String color, double radius) {
super(color);
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public String toString() {
return super.toString() + "圆形,半径为" + radius;
}
}
class Rectangle extends Shape {
protected double length;
protected double width;
public Rectangle(String color, double length, double width) {
super(color);
this.length = length;
this.width = width;
}
@Override
public double getArea() {
return length * width;
}
@Override
public String toString() {
return super.toString() + "矩形,长为" + length + ",宽为" + width;
}
}
class Triangle extends Shape {
protected double a;
protected double b;
protected double c;
public Triangle(String color, double a, double b, double c) {
super(color);
this.a = a;
this.b = b;
this.c = c;
}
@Override
public double getArea() {
double p = (a + b + c) / 2;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
@Override
public String toString() {
return super.toString() + "三角形,边长分别为" + a + "、" + b + "、" + c;
}
}
```
下面是一个简单的测试程序:
```
public class ShapeTest {
public static void main(String[] args) {
Shape s1 = new Circle("红色", 2);
Shape s2 = new Rectangle("黄色", 2, 3);
Shape s3 = new Triangle("蓝色", 3, 4, 5);
System.out.println(s1.toString() + "面积为:" + s1.getArea());
System.out.println(s2.toString() + "面积为:" + s2.getArea());
System.out.println(s3.toString() + "面积为:" + s3.getArea());
}
}
```
运行结果如下:
```
颜色为红色的圆形,半径为2.0面积为:12.566370614359172
颜色为黄色的矩形,长为2.0,宽为3.0面积为:6.0
颜色为蓝色的三角形,边长分别为3.0、4.0、5.0面积为:6.0
```
阅读全文
相关推荐
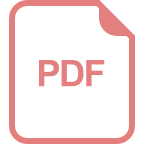

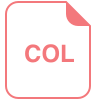
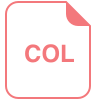
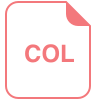
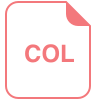
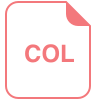
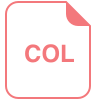
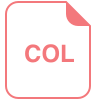
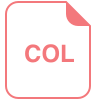
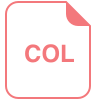
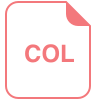
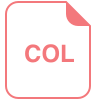
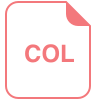
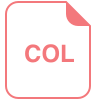
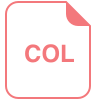
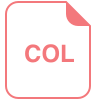