python调用dxgi实时快速截屏
时间: 2023-08-01 08:15:32 浏览: 332
要实现Python调用dxgi进行实时快速截屏,可以使用PyDXGI这个开源工具库,它提供了Python调用dxgi的接口。
下面是一个使用PyDXGI进行实时截屏的简单代码示例:
```python
import pydxgi
# 创建DXGI设备
dxgi_device = pydxgi.create_device()
# 获取DXGI输出
dxgi_output = dxgi_device.get_output()
# 获取DXGI输出的描述
output_desc = dxgi_output.get_desc()
# 获取DXGI输出的位图
bitmap = dxgi_output.get_bitmap()
# 显示位图
bitmap.show()
```
在这个示例中,我们首先创建了一个DXGI设备,然后获取DXGI输出和输出描述。接着,我们获取了DXGI输出的位图,并将其显示出来。
当然,这只是一个简单的示例,你还可以根据自己的需求进行更加复杂的操作,比如将截屏保存为文件,实现全屏幕截屏等。
相关问题
python调用DXGI截图
你可以使用PyDXGI库来调用DXGI进行截图。下面是一个简单的示例代码:
```python
import ctypes
from PIL import Image
# 加载DXGI库
dxgi = ctypes.windll.LoadLibrary('dxgi.dll')
# 创建DXGI工厂
factory = ctypes.POINTER(ctypes.c_void_p)()
dxgi.CreateDXGIFactory(ctypes.byref(ctypes.c_void_p(factory)))
# 获取第一个显示适配器
adapter = ctypes.POINTER(ctypes.c_void_p)()
factory.EnumAdapters(0, ctypes.byref(ctypes.c_void_p(adapter)))
# 获取适配器输出
output = ctypes.POINTER(ctypes.c_void_p)()
adapter.EnumOutputs(0, ctypes.byref(ctypes.c_void_p(output)))
# 获取输出显示模式
mode_desc = ctypes.c_void_p()
output.GetDisplayModeList(dxgi.DXGI_FORMAT_R8G8B8A8_UNORM, 0, ctypes.byref(mode_count), None)
modes = (dxgi.DXGI_MODE_DESC * mode_count.value)()
output.GetDisplayModeList(dxgi.DXGI_FORMAT_R8G8B8A8_UNORM, 0, ctypes.byref(mode_count), modes)
# 获取屏幕资源
resource = ctypes.POINTER(ctypes.c_void_p)()
output.GetResource(ctypes.byref(ctypes.c_void_p(resource)))
# 获取资源表面
surface = ctypes.POINTER(ctypes.c_void_p)()
resource.QueryInterface(ctypes.byref(ctypes.c_void_p(surface)))
# 获取表面描述
desc = ctypes.c_void_p()
surface.GetDesc(ctypes.byref(desc))
# 创建表面表面
surface_data = ctypes.POINTER(ctypes.c_ubyte)()
surface.Map(0x1, ctypes.byref(ctypes.c_void_p(surface_data)))
# 创建图像对象
image_data = (ctypes.c_ubyte * desc.Height * desc.Width * 4)()
ctypes.memmove(ctypes.addressof(image_data), surface_data, ctypes.sizeof(image_data))
# 创建PIL图像
image = Image.frombuffer('RGBA', (desc.Width, desc.Height), image_data, 'raw', 'RGBA', 0, 1)
# 保存图像
image.save('screenshot.png')
# 清理资源
surface.Unmap()
surface.Release()
resource.Release()
output.Release()
adapter.Release()
factory.Release()
```
这段代码使用了PyDXGI库来调用DXGI进行屏幕截图。它首先加载dxgi.dll库,然后创建DXGI工厂。接下来,它获取适配器、输出、显示模式以及屏幕资源。然后,它创建表面表面并获取表面描述。最后,它将表面数据复制到图像对象中,并保存为PNG格式的图像文件。
请注意,这段代码只是一个简单的示例,你可能需要根据你的实际需求进行修改和扩展。另外,确保你已经安装了PyDXGI库和Pillow库(用于处理图像)。
python调用dxgi截图
要在Python中调用DXGI截图,需要使用PyWin32库和Python for .NET库。下面是一个简单的示例代码,可以截取桌面的屏幕截图:
```python
import clr
import win32gui
import win32ui
import win32con
import win32api
import numpy as np
# Load required .NET assemblies
clr.AddReference('System.Drawing')
clr.AddReference('System.Windows.Forms')
from System.Drawing import Bitmap, Graphics
from System.Windows.Forms import Screen
# Get the primary screen
screen = Screen.PrimaryScreen
# Get the screen dimensions
left, top, right, bottom = screen.Bounds.Left, screen.Bounds.Top, screen.Bounds.Right, screen.Bounds.Bottom
# Get the device context handle for the entire screen
hdc_screen = win32gui.GetDC(0)
# Create a memory device context for the screen
hdc_mem = win32gui.CreateCompatibleDC(hdc_screen)
# Create a bitmap to hold the screenshot
bmp_screen = win32ui.CreateBitmap()
bmp_screen.CreateCompatibleBitmap(hdc_screen, right - left, bottom - top)
# Select the bitmap into the memory device context
win32gui.SelectObject(hdc_mem, bmp_screen.GetHandle())
# Copy the screen into the memory device context
win32gui.BitBlt(hdc_mem, 0, 0, right - left, bottom - top, hdc_screen, left, top, win32con.SRCCOPY)
# Convert the bitmap to a numpy array
bmp_array = np.frombuffer(bmp_screen.GetBitmapBits(True), dtype=np.uint8)
bmp_array = bmp_array.reshape((bottom - top, right - left, 4))
# Release the device contexts
win32gui.DeleteObject(bmp_screen.GetHandle())
win32gui.DeleteDC(hdc_mem)
win32gui.ReleaseDC(0, hdc_screen)
# Display the screenshot
import matplotlib.pyplot as plt
plt.imshow(bmp_array)
plt.show()
```
这个示例代码使用了Windows API函数BitBlt将屏幕截图复制到一个内存设备上下文中,然后使用PyWin32库和Python for .NET库将内存设备上下文中的位图转换为一个NumPy数组,最后使用Matplotlib库显示截图。
相关推荐
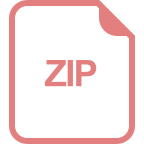












