使用python语言编写一个使PC与三菱PLC通讯的程序
时间: 2024-02-26 13:57:31 浏览: 37
以下是一个简单的使用Python语言编写的程序,可以通过socket与三菱PLC进行通讯:
```python
import socket
# 设置PLC地址和端口号
PLC_IP = '192.168.1.1'
PLC_PORT = 5000
# 创建socket对象
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 连接到PLC
client_socket.connect((PLC_IP, PLC_PORT))
# 发送数据到PLC
send_data = b'D100=1234' # 设置D100的值为1234
client_socket.send(send_data)
# 接收PLC的响应数据
recv_data = client_socket.recv(1024)
print('Received:', recv_data)
# 关闭socket
client_socket.close()
```
在这个程序中,我们使用了Python的socket模块来实现socket通讯。程序首先创建一个socket对象,然后通过connect方法连接到PLC。接着,程序通过send方法发送数据到PLC,并通过recv方法接收PLC的响应数据。最后,程序关闭socket。请注意,这只是一个简单的示例程序,实际的PLC通讯需要更多的代码来处理异常情况和错误处理。
相关问题
编写一个使用Socket与三菱PLC通讯的通讯程序
下面是一个使用Python的Socket库与三菱PLC通讯的简单程序示例,您可以根据自己的实际情况进行修改:
```python
import socket
# PLC的IP地址和端口号
plc_ip = "192.168.0.10"
plc_port = 5000
# 建立Socket连接
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((plc_ip, plc_port))
# 发送数据
send_data = b"\x01\x04\x00\x00\x00\x06\x31\x03\x00\xD0\x00\x01"
client_socket.send(send_data)
# 接收数据
recv_data = client_socket.recv(1024)
print("Received:", recv_data)
# 关闭Socket连接
client_socket.close()
```
该程序使用Socket的TCP连接方式与PLC进行通讯,发送的数据是一个读取D100的命令,接收到的数据是PLC返回的数据。需要注意的是,在编写程序时需要参考三菱PLC通讯协议,正确设置通讯命令和参数。
使用python写一个计算机与三菱PLC通讯的代码
### 回答1:
好的。首先,你需要确保你已经安装了适用于 Python 的三菱 PLC 通讯库,例如 pyMELSEC。
然后,你可以使用以下代码来连接三菱 PLC 并读取一个地址的值:
```
import pyMELSEC
# 连接 PLC
plc = pyMELSEC.PLC()
plc.connect("192.168.0.10", port=8193)
# 读取地址的值
value = plc.read("D100")
# 关闭连接
plc.disconnect()
print(value)
```
在这段代码中,我们使用了 pyMELSEC 库来连接三菱 PLC。首先,我们创建了一个 `PLC` 对象,然后使用 `connect` 方法来连接 PLC。接下来,我们使用 `read` 方法来读取地址 `D100` 的值。最后,我们使用 `disconnect` 方法来断开连接。
希望这些信息对你有帮助。
### 回答2:
要使用Python与三菱PLC通信,可以使用Python的开源库pycomm3。
首先,确保Python已经安装在计算机上,并安装pycomm3库。可以使用以下命令在命令行中进行安装:
```
pip install pycomm3
```
安装完成后,可以编写代码与三菱PLC进行通信。以下是一个简单的示例代码:
```python
from pycomm3 import LogixDriver
# 创建与PLC的连接
with LogixDriver('192.168.1.100') as plc:
# 读取PLC中的D寄存器的值
value = plc.read_tag('D100')
# 将值加1
value += 1
# 将新的值写入PLC中的D寄存器
plc.write_tag('D100', value)
```
如上所示,在代码的开头导入了LogixDriver类。然后,使用`with`语句创建一个与PLC的连接。其中,'192.168.1.100'是PLC的IP地址,可以根据实际情况进行修改。
接下来,在`with`语句中可以使用`read_tag`方法从PLC中读取指定标签(寄存器)的值,并存储在变量中。然后,对这个值进行处理,例如加1。
最后,使用`write_tag`方法将新的值写入到PLC中的指定标签中。
这只是一个简单的示例代码,实际使用中,可能需要根据PLC的具体配置和通信协议进行相应的设置和调整。同时,还可以使用其他方法和功能来实现更复杂的通信操作。
### 回答3:
使用Python编写与三菱PLC通讯的代码可以通过三菱PLC通讯协议进行实现。以下是一个简单的代码示例:
```python
import sys
import pymelsec
# 定义PLC的IP地址和端口号
plc_ip = '192.168.0.1'
plc_port = 6000
# 创建一个连接对象
plc = pymelsec.Melsec(plc_ip, plc_port)
try:
# 连接PLC
plc.open()
# 读取PLC D寄存器的值
data = plc.read_data('D100', 1)
print(f'Read data from PLC D100: {data[0]}')
# 写入PLC D寄存器的值
plc.write_data('D100', 1234)
print('Write data to PLC D100: 1234')
# 读取写入的值
data = plc.read_data('D100', 1)
print(f'Read data from PLC D100: {data[0]}')
except pymelsec.MelsecError as e:
print(f'PLC communication error: {e}')
finally:
# 关闭连接
plc.close()
```
上述代码使用`pymelsec`库实现了Python与三菱PLC的通讯。首先根据PLC的IP地址和端口号创建一个连接对象,通过`open()`方法连接PLC。然后可以使用`read_data()`方法读取PLC指定地址的数据,并使用`write_data()`方法写入数据到PLC指定地址。最后通过`close()`方法关闭连接。
注意,具体的代码实现可能会因PLC型号和通讯协议的不同而有所差异,上述代码仅为示例,具体实现需要根据实际情况进行调整。
相关推荐
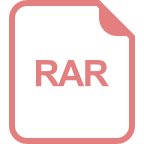












