libcurl 进行SSL认证发送邮件,实际例子使用c++
时间: 2024-11-06 19:16:54 浏览: 20
libcurl是一个流行的开源库,用于在C/C++程序中处理URL并传输数据,包括支持HTTP、HTTPS等协议。如果你需要通过libcurl发送带SSL加密的电子邮件,你可以使用它来构建SMTP连接,并设置相应的认证选项。以下是一个简化的C++示例,展示如何使用libcurl发送带有SSL认证的电子邮件:
```cpp
#include <curl/curl.h>
#include <string>
size_t write_data(void *ptr, size_t size, size_t nmemb, std::string* userp) {
std::string str((char*) ptr, size * nmemb);
*userp += str;
return size * nmemb;
}
void send_email_with_ssl(curl_slist* recipients, const char* smtp_server, const char* username, const char* password) {
CURLcode res;
// 初始化libcurl
curl_global_init(CURL_GLOBAL_DEFAULT);
// 创建一个CURL会话
CURL *curl = curl_easy_init();
if (curl) {
// 设置SMTP服务器地址
curl_easy_setopt(curl, CURLOPT_URL, smtp_server);
// 设置发送邮件的模式
curl_easy_setopt(curl, CURLOPT_MAIL_FROM, "<your-email@example.com>");
// 添加收件人列表
for (auto recipient : recipients) {
curl_easy_setopt(curl, CURLOPT_MAIL_RCPT, recipient);
}
// 设置使用SSL并指定验证信息
curl_easy_setopt(curl, CURLOPT_USE_SSL, (long)CURLUSESSL_ALL); // 使用SSL/TLS
curl_easy_setopt(curl, CURLOPT_USERNAME, username);
curl_easy_setopt(curl, CURLOPT_PASSWORD, password);
// 设置POST请求头
struct curl_slist* headers = NULL;
headers = curl_slist_append(headers, "Content-Type: text/plain; charset=UTF-8");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
// 设置数据写入函数,这里只是一个简单的示例,实际上应构造邮件内容
std::string mail_content = "Hello from libcurl!";
curl_easy_setopt(curl, CURLOPT_READFUNCTION, write_data);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &mail_content);
// 执行请求
res = curl_easy_perform(curl);
// 清理
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
}
curl_slist_free_all(headers);
curl_easy_cleanup(curl);
// 关闭libcurl
curl_global_cleanup();
}
}
// 示例:添加收件人地址到链表
std::string to_string_list(const std::vector<std::string>& recipients) {
std::string result = "";
for (const auto& email : recipients) {
result += email + ", ";
}
return result.substr(0, result.size() - 2); // 去掉最后一个逗号
}
int main() {
std::vector<std::string> recipients = {"recipient1@example.com", "recipient2@example.com"};
std::string smtp_server = "smtp.example.com";
const char* username = "your_username";
const char* password = "your_password";
// 创建收件人链表
curl_slist* recipients_list = nullptr;
recipients_list = curl_slist_append(recipients_list, recipients[0].c_str());
for (size_t i = 1; i < recipients.size(); ++i) {
recipients_list = curl_slist_append(recipients_list, recipients[i].c_str());
}
// 发送邮件
send_email_with_ssl(recipients_list, smtp_server, username, password);
// 清理链表
curl_slist_free_all(recipients_list);
return 0;
}
```
阅读全文
相关推荐
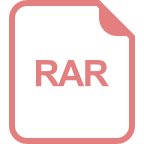
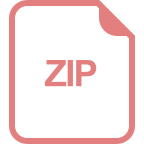
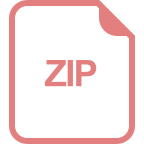

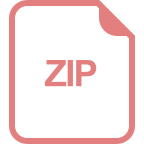
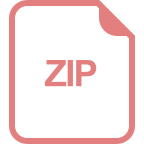
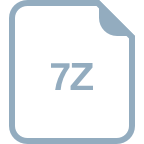
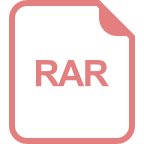
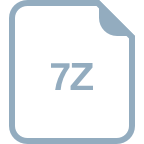
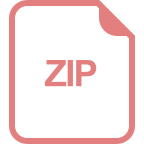
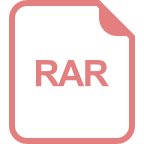
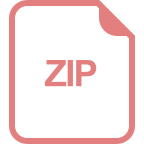
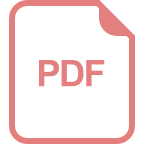
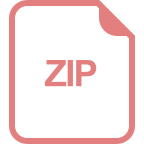
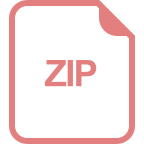
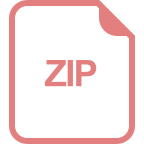
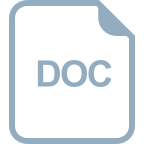
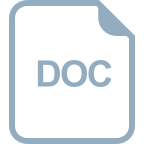
