帮我用libcurl写一个带证书访问https://www.baidu.com的c++的get和post封装访问的例子
时间: 2024-01-24 08:17:58 浏览: 227
好的,以下是一个简单的使用libcurl进行HTTPS GET和POST请求的C++代码示例:
```c++
#include <iostream>
#include <curl/curl.h>
// HTTPS GET请求的回调函数
size_t curl_callback_get(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
// HTTPS POST请求的回调函数
size_t curl_callback_post(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
// HTTPS GET请求封装函数
std::string https_get(const std::string& url, const std::string& cert_path) {
std::string response;
CURL* curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_CAINFO, cert_path.c_str());
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, true);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, curl_callback_get);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl);
}
return response;
}
// HTTPS POST请求封装函数
std::string https_post(const std::string& url, const std::string& cert_path, const std::string& post_fields) {
std::string response;
CURL* curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_CAINFO, cert_path.c_str());
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, true);
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, post_fields.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, curl_callback_post);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl);
}
return response;
}
int main() {
// HTTPS GET请求示例
std::string url = "https://www.baidu.com/";
std::string cert_path = "/path/to/your/certificate.pem";
std::string response = https_get(url, cert_path);
std::cout << "Response from " << url << ":\n" << response << std::endl;
// HTTPS POST请求示例
url = "https://www.example.com/";
cert_path = "/path/to/your/certificate.pem";
std::string post_fields = "foo=bar&baz=qux";
response = https_post(url, cert_path, post_fields);
std::cout << "Response from " << url << ":\n" << response << std::endl;
return 0;
}
```
需要注意的是,要使用HTTPS协议,需要提供证书文件路径,并开启SSL验证选项。以上代码中使用了两个回调函数`curl_callback_get`和`curl_callback_post`,分别用于处理HTTPS GET和POST请求的响应结果。
另外,需要在编译时链接libcurl库,例如使用以下命令编译:
```
g++ -o main main.cpp -lcurl
```
阅读全文
相关推荐
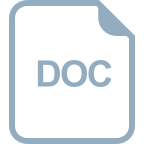
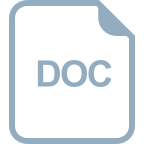
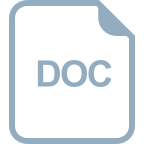





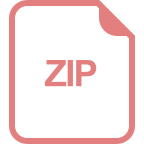
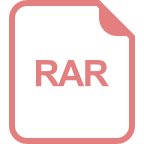






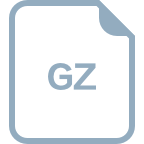

