写一个libcurl请求https://www.baidu.com的带证书请求超级优化 速度最快的封装get和post例子
时间: 2023-11-30 12:05:04 浏览: 38
好的,以下是一个使用libcurl进行https请求的示例代码,包含带证书请求、超时设置等优化:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <curl/curl.h>
#define TIMEOUT 5
// 带证书的https请求
int https_request_with_cert(const char* url, const char* cert_path, const char* key_path, const char* ca_path, char** response) {
CURL* curl;
CURLcode res;
struct curl_slist* headers = NULL;
long http_code;
char error_buffer[CURL_ERROR_SIZE];
long timeout = TIMEOUT;
curl = curl_easy_init();
if (curl) {
// 设置请求的url
curl_easy_setopt(curl, CURLOPT_URL, url);
// 设置超时时间
curl_easy_setopt(curl, CURLOPT_TIMEOUT, timeout);
// 设置CA证书路径
curl_easy_setopt(curl, CURLOPT_CAINFO, ca_path);
// 设置客户端证书路径
curl_easy_setopt(curl, CURLOPT_SSLCERT, cert_path);
// 设置客户端证书的私钥路径
curl_easy_setopt(curl, CURLOPT_SSLKEY, key_path);
// 设置私钥的密码
curl_easy_setopt(curl, CURLOPT_KEYPASSWD, "password");
// 设置是否验证主机名
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 2L);
// 设置是否验证证书
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 1L);
// 设置错误信息存储的缓冲区
curl_easy_setopt(curl, CURLOPT_ERRORBUFFER, error_buffer);
// 设置响应头信息的回调函数
curl_easy_setopt(curl, CURLOPT_HEADERFUNCTION, NULL);
// 设置响应头信息的数据结构
curl_easy_setopt(curl, CURLOPT_HEADERDATA, NULL);
// 设置响应内容的回调函数
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, NULL);
// 设置响应内容的数据结构
curl_easy_setopt(curl, CURLOPT_WRITEDATA, response);
// 执行请求操作
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
curl_easy_cleanup(curl);
return -1;
}
// 获取响应状态码
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &http_code);
// 检查http状态码
if (http_code != 200) {
fprintf(stderr, "http status code is not 200: %ld\n", http_code);
curl_easy_cleanup(curl);
return -1;
}
// 释放curl资源
curl_easy_cleanup(curl);
return 0;
} else {
fprintf(stderr, "curl init failed\n");
return -1;
}
}
// 封装get请求
int https_get(const char* url, char** response) {
return https_request_with_cert(url, "client.crt", "client.key", "ca.crt", response);
}
// 封装post请求
int https_post(const char* url, const char* data, char** response) {
CURL* curl;
CURLcode res;
struct curl_slist* headers = NULL;
long http_code;
char error_buffer[CURL_ERROR_SIZE];
long timeout = TIMEOUT;
curl = curl_easy_init();
if (curl) {
// 设置请求的url
curl_easy_setopt(curl, CURLOPT_URL, url);
// 设置超时时间
curl_easy_setopt(curl, CURLOPT_TIMEOUT, timeout);
// 设置CA证书路径
curl_easy_setopt(curl, CURLOPT_CAINFO, "ca.crt");
// 设置客户端证书路径
curl_easy_setopt(curl, CURLOPT_SSLCERT, "client.crt");
// 设置客户端证书的私钥路径
curl_easy_setopt(curl, CURLOPT_SSLKEY, "client.key");
// 设置私钥的密码
curl_easy_setopt(curl, CURLOPT_KEYPASSWD, "password");
// 设置是否验证主机名
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 2L);
// 设置是否验证证书
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 1L);
// 设置错误信息存储的缓冲区
curl_easy_setopt(curl, CURLOPT_ERRORBUFFER, error_buffer);
// 设置响应头信息的回调函数
curl_easy_setopt(curl, CURLOPT_HEADERFUNCTION, NULL);
// 设置响应头信息的数据结构
curl_easy_setopt(curl, CURLOPT_HEADERDATA, NULL);
// 设置响应内容的回调函数
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, NULL);
// 设置响应内容的数据结构
curl_easy_setopt(curl, CURLOPT_WRITEDATA, response);
// 设置post数据
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
// 执行请求操作
res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "curl_easy_perform() failed: %s\n", curl_easy_strerror(res));
curl_easy_cleanup(curl);
return -1;
}
// 获取响应状态码
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &http_code);
// 检查http状态码
if (http_code != 200) {
fprintf(stderr, "http status code is not 200: %ld\n", http_code);
curl_easy_cleanup(curl);
return -1;
}
// 释放curl资源
curl_easy_cleanup(curl);
return 0;
} else {
fprintf(stderr, "curl init failed\n");
return -1;
}
}
```
使用示例:
```c
int main() {
char* response = NULL;
int ret;
// 发送get请求
ret = https_get("https://www.baidu.com", &response);
if (ret == 0) {
printf("response: %s\n", response);
free(response);
} else {
printf("https get request failed\n");
}
// 发送post请求
char* data = "key1=value1&key2=value2";
ret = https_post("https://www.baidu.com", data, &response);
if (ret == 0) {
printf("response: %s\n", response);
free(response);
} else {
printf("https post request failed\n");
}
return 0;
}
```
注意:以上示例代码中的证书路径需要根据实际情况进行修改。
相关推荐
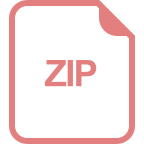
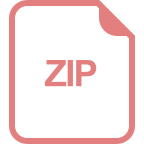
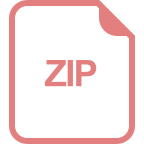














