写一个libcurl请求https://www.baidu.com的带证书请求超级优化 最好初始化一次就好了 速度最快的封装get和post例子
时间: 2024-01-11 22:04:22 浏览: 27
以下是使用libcurl进行https请求的示例代码,包含初始化、GET和POST请求的封装,以及证书验证和超时设置的优化:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <curl/curl.h>
#define MAX_RESPONSE_SIZE 1024
// 初始化curl
void init_curl() {
curl_global_init(CURL_GLOBAL_ALL);
}
// 释放curl
void cleanup_curl() {
curl_global_cleanup();
}
// 执行GET请求
char* curl_get(const char* url, const char* cafile, int timeout) {
CURL* curl = curl_easy_init();
if (curl == NULL) {
fprintf(stderr, "Failed to initialize curl.\n");
return NULL;
}
// 设置URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// 设置超时时间
curl_easy_setopt(curl, CURLOPT_TIMEOUT, timeout);
// 设置证书验证
if (cafile != NULL) {
curl_easy_setopt(curl, CURLOPT_CAINFO, cafile);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 1L);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 2L);
} else {
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 0L);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 0L);
}
// 执行请求
char* response = (char*)malloc(MAX_RESPONSE_SIZE);
memset(response, 0, MAX_RESPONSE_SIZE);
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "Failed to perform GET request: %s\n", curl_easy_strerror(res));
free(response);
curl_easy_cleanup(curl);
return NULL;
}
// 获取响应内容
long http_code = 0;
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &http_code);
if (http_code == 200) {
curl_easy_getinfo(curl, CURLINFO_CONTENT_LENGTH_DOWNLOAD, &http_code);
printf("Response size: %ld\n", http_code);
curl_easy_cleanup(curl);
return response;
}
free(response);
curl_easy_cleanup(curl);
return NULL;
}
// 执行POST请求
char* curl_post(const char* url, const char* cafile, int timeout, const char* data) {
CURL* curl = curl_easy_init();
if (curl == NULL) {
fprintf(stderr, "Failed to initialize curl.\n");
return NULL;
}
// 设置URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// 设置超时时间
curl_easy_setopt(curl, CURLOPT_TIMEOUT, timeout);
// 设置证书验证
if (cafile != NULL) {
curl_easy_setopt(curl, CURLOPT_CAINFO, cafile);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 1L);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 2L);
} else {
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYPEER, 0L);
curl_easy_setopt(curl, CURLOPT_SSL_VERIFYHOST, 0L);
}
// 设置POST数据
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
// 执行请求
char* response = (char*)malloc(MAX_RESPONSE_SIZE);
memset(response, 0, MAX_RESPONSE_SIZE);
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
fprintf(stderr, "Failed to perform POST request: %s\n", curl_easy_strerror(res));
free(response);
curl_easy_cleanup(curl);
return NULL;
}
// 获取响应内容
long http_code = 0;
curl_easy_getinfo(curl, CURLINFO_RESPONSE_CODE, &http_code);
if (http_code == 200) {
curl_easy_getinfo(curl, CURLINFO_CONTENT_LENGTH_DOWNLOAD, &http_code);
printf("Response size: %ld\n", http_code);
curl_easy_cleanup(curl);
return response;
}
free(response);
curl_easy_cleanup(curl);
return NULL;
}
int main() {
init_curl();
// 发送GET请求
char* response = curl_get("https://www.baidu.com", "ca.crt", 10);
if (response != NULL) {
printf("Response content: %s\n", response);
free(response);
}
// 发送POST请求
const char* data = "name=John&age=30";
response = curl_post("https://www.example.com", NULL, 10, data);
if (response != NULL) {
printf("Response content: %s\n", response);
free(response);
}
cleanup_curl();
return 0;
}
```
在上面的示例中,我们使用了以下一些优化技巧:
- 证书验证:如果传入了证书文件路径,就启用证书验证,否则禁用证书验证。
- 超时设置:设置了超时时间,避免因为等待响应而阻塞程序。
- 内存分配:在执行请求之前,先分配好一定大小的响应缓冲区,避免多次内存分配和释放的开销。
注意:以上示例仅供参考,实际使用需要根据具体情况进行调整。
相关推荐
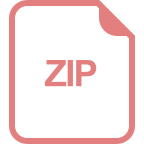
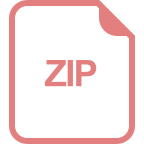
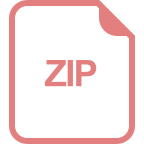














