java弹窗表格把文件的书存入表格实现管理员对书籍的添加和删除的代码,不用数据库
时间: 2024-03-16 18:46:29 浏览: 15
以下是一个使用Java Swing实现弹窗表格的示例代码,可以实现管理员对书籍的添加和删除,数据存储在内存中的数据结构中,而不是使用数据库。
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
public class BookManagerDialog extends JDialog {
private static final long serialVersionUID = 1L;
private List<Book> bookList = new ArrayList<>();
private JTable table;
private DefaultTableModel model;
public BookManagerDialog(JFrame parent) {
super(parent, true);
setTitle("图书管理");
setSize(400, 300);
setLocationRelativeTo(null);
// 表格模型
model = new DefaultTableModel();
model.addColumn("书名");
model.addColumn("作者");
model.addColumn("出版社");
table = new JTable(model);
// 添加滚动条
JScrollPane scrollPane = new JScrollPane(table);
// 添加按钮
JButton addButton = new JButton("添加");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addBook();
}
});
JButton deleteButton = new JButton("删除");
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteBook();
}
});
JPanel buttonPanel = new JPanel();
buttonPanel.add(addButton);
buttonPanel.add(deleteButton);
// 添加到对话框中
getContentPane().add(scrollPane, BorderLayout.CENTER);
getContentPane().add(buttonPanel, BorderLayout.SOUTH);
}
public void addBook() {
BookFormDialog formDialog = new BookFormDialog(this);
formDialog.setVisible(true);
if (formDialog.isSubmit()) {
Book book = new Book(formDialog.getBookName(),
formDialog.getAuthorName(),
formDialog.getPublisherName());
bookList.add(book);
model.addRow(new Object[] {book.getName(),
book.getAuthor(), book.getPublisher()});
}
}
public void deleteBook() {
int selectedRow = table.getSelectedRow();
if (selectedRow >= 0) {
bookList.remove(selectedRow);
model.removeRow(selectedRow);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("测试");
frame.setSize(800, 600);
frame.setLocationRelativeTo(null);
BookManagerDialog dialog = new BookManagerDialog(frame);
dialog.setVisible(true);
}
}
class BookFormDialog extends JDialog {
private static final long serialVersionUID = 1L;
private boolean submit;
private String bookName;
private String authorName;
private String publisherName;
public BookFormDialog(JDialog parent) {
super(parent, true);
setTitle("添加图书");
setSize(400, 300);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
getContentPane().add(panel, BorderLayout.CENTER);
panel.setLayout(null);
// 添加表单元素
JLabel bookNameLabel = new JLabel("书名:");
bookNameLabel.setBounds(10, 20, 60, 25);
panel.add(bookNameLabel);
JTextField bookNameField = new JTextField();
bookNameField.setBounds(80, 20, 200, 25);
panel.add(bookNameField);
JLabel authorNameLabel = new JLabel("作者:");
authorNameLabel.setBounds(10, 50, 60, 25);
panel.add(authorNameLabel);
JTextField authorNameField = new JTextField();
authorNameField.setBounds(80, 50, 200, 25);
panel.add(authorNameField);
JLabel publisherNameLabel = new JLabel("出版社:");
publisherNameLabel.setBounds(10, 80, 60, 25);
panel.add(publisherNameLabel);
JTextField publisherNameField = new JTextField();
publisherNameField.setBounds(80, 80, 200, 25);
panel.add(publisherNameField);
// 添加按钮
JButton okButton = new JButton("确定");
okButton.setBounds(50, 150, 80, 25);
okButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
bookName = bookNameField.getText();
authorName = authorNameField.getText();
publisherName = publisherNameField.getText();
submit = true;
setVisible(false);
}
});
panel.add(okButton);
JButton cancelButton = new JButton("取消");
cancelButton.setBounds(150, 150, 80, 25);
cancelButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
submit = false;
setVisible(false);
}
});
panel.add(cancelButton);
}
public boolean isSubmit() {
return submit;
}
public String getBookName() {
return bookName;
}
public String getAuthorName() {
return authorName;
}
public String getPublisherName() {
return publisherName;
}
}
class Book {
private String name;
private String author;
private String publisher;
public Book(String name, String author, String publisher) {
this.name = name;
this.author = author;
this.publisher = publisher;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
}
```
说明:
1. BookManagerDialog类是弹窗表格的主界面,使用JTable实现表格,使用DefaultTableModel作为表格模型,将数据存储在bookList中,通过添加和删除按钮修改表格数据。
2. BookFormDialog类是添加图书的表单弹窗,使用JTextField作为表单元素,通过getXXX()方法获取用户输入的数据,通过isSubmit()方法判断用户是否点击了“确定”按钮。
3. Book类是一个简单的数据模型类,用于存储图书信息。
相关推荐
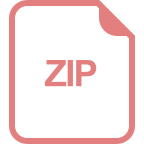
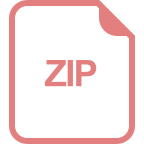
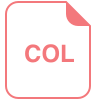
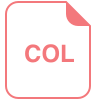
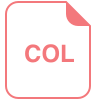
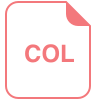
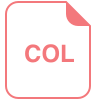









