std::vector的find
时间: 2023-12-14 15:05:55 浏览: 76
std::vector的find函数是用来在vector中查找指定元素的,其函数原型为:
```c++
iterator find (const value_type& val);
```
其中,val为要查找的元素值,函数返回一个迭代器,指向第一个等于val的元素。如果没有找到,则返回vector的end迭代器。
例如,下面的代码演示了如何使用find函数在vector中查找指定元素:
```c++
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = vec.find(3);
if (it != vec.end()) {
std::cout << "Found element: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
输出结果为:
```
Found element: 3
```
相关问题
std::vector find
std::vector 的 find 函数是用来在 vector 容器中查找指定元素的,其函数原型为:
```c++
iterator find (const value_type& val);
```
其中,val 为要查找的元素值,函数返回一个迭代器,指向第一个等于 val 的元素,如果没有找到,则返回指向 vector 容器尾部的迭代器 end()。
例如,下面的代码演示了如何使用 find 函数在 vector 容器中查找指定元素:
```c++
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = vec.find(3);
if (it != vec.end()) {
std::cout << "Found element: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
```
输出结果为:
```
Found element: 3
```
std::find 返回在std::vector中的位置
`std::find` 是 C++ 标准库中的一个算法,用于在容器中查找指定元素。当在 `std::vector` 中使用 `std::find` 时,它会返回一个迭代器,指向找到的元素的位置。如果没有找到该元素,则返回指向容器末尾的迭代器(即 `end()`)。
示例代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int target = 3;
// 使用 std::find 查找目标元素
std::vector<int>::iterator it = std::find(vec.begin(), vec.end(), target);
if (it != vec.end()) {
std::cout << "找到元素 " << target << ",位置为:" << (it - vec.begin()) << std::endl;
} else {
std::cout << "未找到元素 " << target << std::endl;
}
return 0;
}
```
在这个例子中,`std::find` 在 `vec` 中查找元素 `3`,并返回一个指向该元素的迭代器。通过计算 `(it - vec.begin())`,我们可以得到元素在 `std::vector` 中的位置。
阅读全文
相关推荐
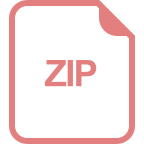





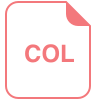







