matlab实现决策树算法
时间: 2023-07-11 14:51:40 浏览: 152
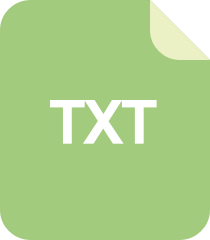
决策树算法
以下是使用MATLAB实现决策树算法的一般步骤:
1. 准备数据集:通过读取数据文件或手动输入数据,准备训练数据集。
2. 数据预处理:对数据集进行清洗、去重、缺失值处理等操作。
3. 特征选择:通过某些特征选择算法选出最优的特征作为划分属性。
4. 树的生成:采用ID3、C4.5、CART等算法生成决策树。
5. 决策树的剪枝:对已生成的决策树进行剪枝以避免过拟合。
6. 模型评估:使用测试数据集对生成的决策树模型进行评估。
以下是使用MATLAB实现决策树算法的示例代码:
```matlab
% 准备数据集
data = [1, 1, 1;
1, 1, 0;
0, 1, 1;
0, 0, 1;
0, 0, 0;
1, 0, 0;
1, 0, 1;
0, 1, 0];
% 准备标签
labels = [1, 1, -1, -1, -1, 1, 1, -1];
% 生成决策树
tree = fitctree(data, labels);
% 预测新数据
new_data = [1, 0, 1];
[~, score] = predict(tree, new_data);
disp(score(2)); % 输出预测结果
```
在以上示例代码中,我们首先准备了一个数据集和标签,然后使用`fitctree`函数生成了决策树模型。最后,我们使用`predict`函数对新的数据进行预测,输出了预测结果。
阅读全文
相关推荐
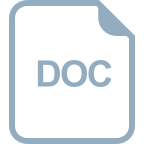
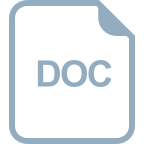
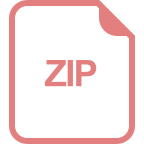

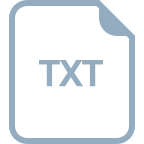
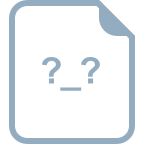
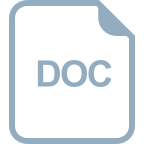
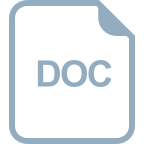
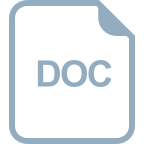
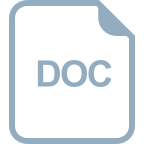

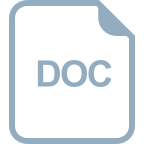
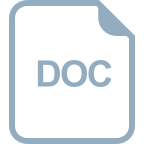
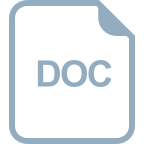