文档提取集合后怎么构建倒排索引
时间: 2024-05-22 07:12:11 浏览: 11
构建倒排索引需要以下步骤:
1. 遍历文档提取集合,对于每个文档,提取出其中的关键词或者特征。
2. 对于每个关键词或者特征,记录它出现在哪些文档中。
3. 将文档和关键词或者特征的对应关系保存在倒排索引中。
4. 倒排索引的结构通常是一个哈希表,每个关键词或者特征是一个键,对应的值是一个文档列表。
5. 在查询时,根据查询关键词或者特征在倒排索引中查找对应的文档列表。
6. 可以使用压缩算法等技术来优化倒排索引的存储和查询效率。
总之,构建倒排索引需要将文档提取集合中的关键词或者特征与文档进行对应,然后将这种对应关系保存在倒排索引中,以便在查询时可以快速找到与查询关键词或者特征相关的文档。
相关问题
请给我一个提取文档集合后构建倒排索引的代码
这是一个Python的示例代码,用于提取文档集合并构建倒排索引:
```python
import os
import re
# 定义一个函数用于提取文档集合并返回一个字典
def extract_docs(dir_path):
docs = {}
for filename in os.listdir(dir_path):
if filename.endswith(".txt"):
with open(os.path.join(dir_path, filename), "r", encoding="utf-8") as f:
content = f.read()
docs[filename] = content
return docs
# 定义一个函数用于构建倒排索引并返回一个字典
def build_inverted_index(docs):
inverted_index = {}
for doc_id, doc_content in docs.items():
words = re.findall(r'\b\w+\b', doc_content.lower())
for word in words:
if word not in inverted_index:
inverted_index[word] = {doc_id: 1}
elif doc_id not in inverted_index[word]:
inverted_index[word][doc_id] = 1
else:
inverted_index[word][doc_id] += 1
return inverted_index
# 使用示例
docs = extract_docs("path/to/document/collection")
inverted_index = build_inverted_index(docs)
print(inverted_index)
```
该代码将读取指定目录下的所有以“.txt”为后缀的文件,并将它们保存到一个字典中,其中键为文件名,值为文件内容。然后,它将构建一个倒排索引,该索引将单词映射到包含该单词的文档列表。输出结果将是一个字典,其中键为单词,值为字典,该字典将文档ID映射到该单词在该文档中出现的次数。
doris的倒排索引
Doris的倒排索引是一种用于快速检索文档的数据结构。倒排索引(Inverted Index)是指以单词作为索引的关键词表,通过将文档中的单词进行提取和归纳,建立起单词与文档之间的映射关系。
在Doris中,倒排索引通常用于支持全文搜索功能。它将文档集合中的每个单词与包含该单词的文档进行关联,存储为一个键值对。这样,当用户搜索某个单词时,系统可以通过倒排索引快速找到包含该单词的文档。
倒排索引的构建过程一般包括以下步骤:
1. 预处理:对文档进行分词和过滤等操作,将文档转化为单词序列。
2. 建立倒排表:将每个单词与包含它的文档进行映射,形成倒排表。
3. 索引优化:对倒排表进行压缩和优化,提高检索效率。
4. 搜索处理:根据用户查询的关键词,在倒排索引中查找相应的文档。
Doris使用倒排索引来支持高效的文本搜索和过滤功能,通过快速定位相关文档,提升用户的搜索体验。
相关推荐
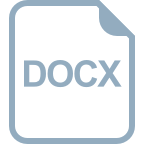
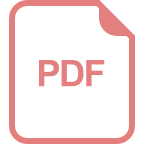
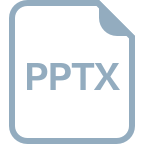







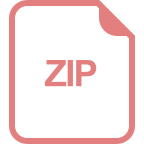
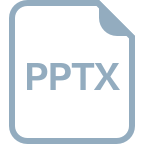
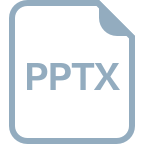
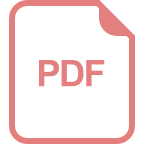
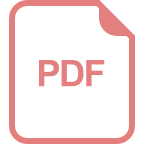
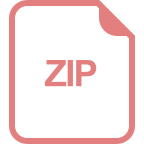