c++ string malloc
时间: 2023-09-03 21:09:38 浏览: 41
你可以使用`malloc`函数在C语言中动态分配内存空间来创建字符串。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char* str = (char*) malloc(10 * sizeof(char)); // 分配10个字符的空间
if (str == NULL) {
printf("内存分配失败");
return 1;
}
strcpy(str, "Hello");
printf("%s\n", str);
相关问题
c++ string乘法
在C语言中,对字符串进行乘法运算可以通过循环和字符串拼接来实现。要实现字符串乘法,首先需要定义一个函数,接受两个字符串和一个整数作为参数,实现将一个字符串重复指定次数的功能。
具体的实现过程可以如下所示:
```c
#include <stdio.h>
#include <string.h>
char* stringMultiply(const char* str, int n) {
int len = strlen(str);
char* result = (char*)malloc(len * n + 1);
result[0] = '\0'; // 初始化为空字符串
for (int i = 0; i < n; i++) {
strcat(result, str); // 将str拼接到result中
}
return result;
}
int main() {
char* str1 = "Hello";
int n = 3;
char* multipliedStr = stringMultiply(str1, n);
printf("Result: %s\n", multipliedStr);
free(multipliedStr); // 释放内存
return 0;
}
```
在上面的示例中,我们定义了一个stringMultiply函数,它接受一个字符串和一个整数作为参数,将字符串重复指定次数后返回。在main函数中,我们调用了stringMultiply函数将字符串"Hello"重复3次后输出结果。
通过这种方式,我们就可以在C语言中实现字符串的乘法操作。
c++ string 切片索引
在C语言中,可以使用指针和数组下标来实现字符串切片索引。
例如,假设有以下字符串:
```c
char str[] = "Hello, world!";
```
要获取索引为2到6的子字符串(包括2和6),可以使用以下代码:
```c
char *substr = malloc(6 * sizeof(char)); // 分配6个字符的空间
substr[5] = '\0'; // 在最后一个字符后面加上'\0',以便将其视为字符串
for (int i = 0; i < 5; i++) {
substr[i] = str[i+2];
}
printf("%s\n", substr); // 输出 "llo, "
```
这里使用了指针和数组下标来实现字符串切片索引。首先使用`malloc()`函数分配了6个字符的空间,然后使用循环和数组下标将原字符串中索引为2到6的字符复制到新的字符串中。最后,在新字符串的最后一个字符后面添加了一个`\0`字符,以便将其视为字符串。最后输出新字符串即可。
相关推荐
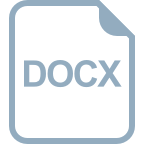
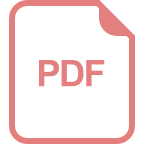
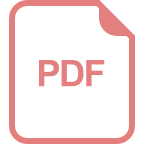












