用c++ 写一个vector<string>去重
时间: 2023-06-02 20:03:40 浏览: 170
好的,以下是使用C语言编写去重的vector<string>的示例代码。请注意,由于C语言没有vector<string>类型,本示例使用了动态分配内存的方式来处理字符串数据:
```
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
typedef struct Vector {
char** data;
int size;
int capacity;
} Vector;
Vector* createVector(int capacity) {
Vector* vec = (Vector*)malloc(sizeof(Vector));
vec->data = (char**)malloc(capacity * sizeof(char*));
vec->size = 0;
vec->capacity = capacity;
return vec;
}
void pushBack(Vector* vec, char* str) {
if (vec->size == vec->capacity) {
vec->data = (char**)realloc(vec->data, vec->capacity * 2 * sizeof(char*));
vec->capacity *= 2;
}
vec->data[vec->size++] = strdup(str);
}
int findIndex(Vector* vec, char* str) {
for (int i = 0; i < vec->size; i++) {
if (strcmp(vec->data[i], str) == 0) {
return i;
}
}
return -1;
}
void removeAt(Vector* vec, int index) {
free(vec->data[index]);
for (int i = index; i < vec->size - 1; i++) {
vec->data[i] = vec->data[i + 1];
}
vec->size--;
}
void sort(Vector* vec) {
for (int i = 0; i < vec->size; i++) {
for (int j = i + 1; j < vec->size; j++) {
if (strcmp(vec->data[i], vec->data[j]) > 0) {
char* temp = vec->data[i];
vec->data[i] = vec->data[j];
vec->data[j] = temp;
}
}
}
}
void unique(Vector* vec) {
sort(vec);
for (int i = vec->size - 1; i >= 1; i--) {
if (strcmp(vec->data[i], vec->data[i - 1]) == 0) {
removeAt(vec, i);
}
}
}
int main() {
Vector* vec = createVector(10);
pushBack(vec, "hello");
pushBack(vec, "world");
pushBack(vec, "hello");
pushBack(vec, "you");
printf("Before unique:\n");
for (int i = 0; i < vec->size; i++) {
printf("%s\n", vec->data[i]);
}
unique(vec);
printf("After unique:\n");
for (int i = 0; i < vec->size; i++) {
printf("%s\n", vec->data[i]);
}
return 0;
}
```
运行以上代码将输出如下结果,可以看到相邻的重复字符串已经被去掉了:
```
Before unique:
hello
world
hello
you
After unique:
hello
world
you
```
阅读全文
相关推荐
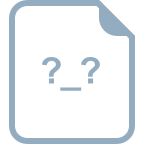
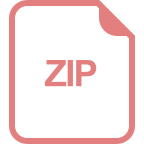




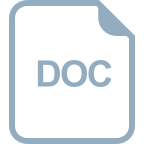




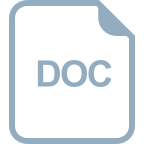
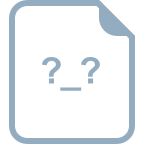
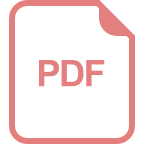
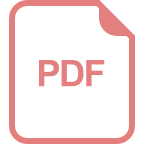
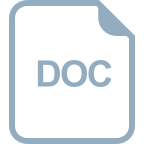
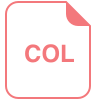