使用C#语言,架构一个包含视图层,业务逻辑和数据访问层的三层架构WinForm,或者Asp.Net或者WPF项目,使用查询命令,对指定数据库做一些数据展示
时间: 2024-10-07 14:05:09 浏览: 49
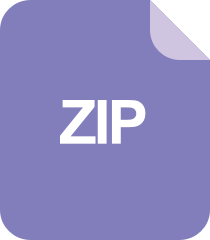
餐饮管理系统源码 餐饮茶楼管理系统 C# winform源码asp.net.zip

在C#中构建一个三层架构的项目通常涉及MVC(Model-View-Controller)设计模式,对于WinForms、ASP.NET或WPF项目,你可以按照以下步骤进行:
1. **模型层(Model)**:
- 定义数据实体类(Data Model),它们代表数据库中的表结构,例如`Customer`或`Order`。使用Entity Framework (EF)、ADO.NET等库来操作数据库。
```csharp
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
// 其他字段...
}
```
2. **数据访问层(Data Access Layer, DAL)**:
- 创建一个单独的层,用于处理所有数据库交互。使用Entity Framework DbContext,编写Repository Pattern的接口或类。
```csharp
public interface ICustomerRepository
{
List<Customer> GetAll();
Customer GetById(int id);
// 添加、更新和删除操作...
}
public class CustomerRepository : ICustomerRepository
{
private readonly DbContext _dbContext;
public CustomerRepository(DbContext dbContext)
{
_dbContext = dbContext;
}
public List<Customer> GetAll()
{
return _dbContext.Customers.ToList();
}
}
```
3. **业务逻辑层(Business Logic Layer, BLL)**:
- 这里处理数据验证、转换和处理复杂逻辑。创建服务类如`CustomerService`,它依赖于数据访问层。
```csharp
public class CustomerService
{
private readonly ICustomerRepository _customerRepository;
public CustomerService(ICustomerRepository customerRepository)
{
_customerRepository = customerRepository;
}
public IEnumerable<Customer> GetFilteredCustomers(string searchTerm)
{
return _customerRepository.GetAll().Where(c => c.Name.Contains(searchTerm));
}
}
```
4. **视图层(View)**:
- 对于WinForms,可以是Windows Forms控件的布局;对于ASP.NET,可能是Web页面上的GridView;WPF则是UserControl或Window。
- WinForms: 使用DataGridView展示查询结果,并绑定到`Customer`列表。
- ASP.NET MVC: 返回一个包含查询结果的ActionResult,将数据绑定到 Razor 视图的GridView或其他控件上。
- WPF: 通过DataGrid或CollectionViewSource将数据绑定到UI元素。
**相关问题--:**
1. 如何在三层架构中引入事务管理?
2. 如何在BLL中封装复杂的数据库查询?
3. 数据访问层如何处理数据库连接池?
阅读全文
相关推荐
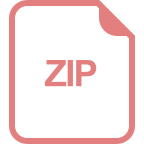
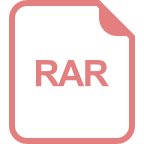
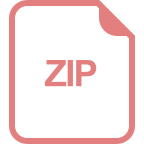
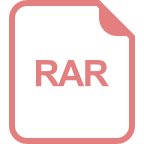
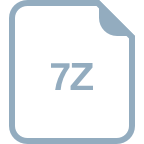
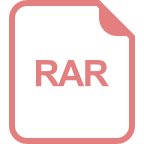
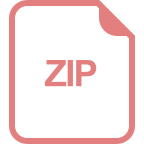
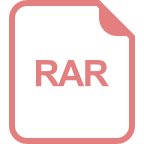
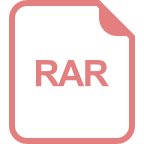
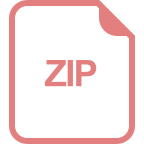
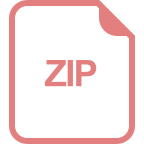
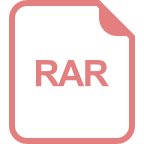
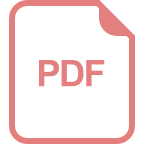
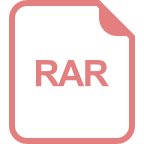
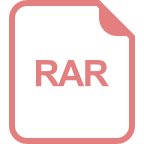
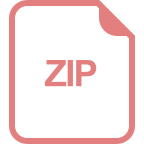
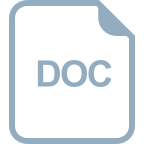
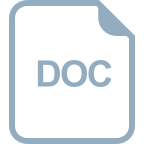