Android 脱离activity 实现一个单独全局侧滑应用
时间: 2024-05-02 09:23:10 浏览: 160
要脱离Activity实现一个单独全局侧滑应用,可以使用Android中的Service来实现。Service是一种在后台运行的组件,它可以独立于Activity运行,并且可以在后台处理一些耗时的任务,如网络请求等。
具体实现步骤如下:
1. 创建一个Service类,并在onCreate方法中初始化全局侧滑菜单布局,并将其添加到WindowManager中。
```java
public class SlideMenuService extends Service {
private WindowManager mWindowManager;
private View mSlideMenuView;
@Override
public void onCreate() {
super.onCreate();
// 初始化全局侧滑菜单布局
mSlideMenuView = LayoutInflater.from(this).inflate(R.layout.slide_menu_layout, null);
// 将全局侧滑菜单布局添加到WindowManager中
mWindowManager = (WindowManager) getSystemService(WINDOW_SERVICE);
WindowManager.LayoutParams layoutParams = new WindowManager.LayoutParams();
layoutParams.type = WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY;
layoutParams.format = PixelFormat.TRANSLUCENT;
layoutParams.gravity = Gravity.LEFT | Gravity.CENTER_VERTICAL;
layoutParams.width = WindowManager.LayoutParams.WRAP_CONTENT;
layoutParams.height = WindowManager.LayoutParams.MATCH_PARENT;
mWindowManager.addView(mSlideMenuView, layoutParams);
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onDestroy() {
super.onDestroy();
// 从WindowManager中移除全局侧滑菜单布局
mWindowManager.removeView(mSlideMenuView);
}
}
```
2. 在AndroidManifest.xml文件中注册Service。
```xml
<service android:name=".SlideMenuService" />
```
3. 在需要使用全局侧滑菜单的地方启动Service。
```java
Intent intent = new Intent(MainActivity.this, SlideMenuService.class);
startService(intent);
```
需要注意的是,由于Android 8.0及以上版本限制了应用的后台服务,如果想要在后台运行Service,需要使用startForeground方法将Service变成前台服务,并且需要显示一个通知,告诉用户Service正在运行。
完整代码可以参考以下示例:
```java
public class MainActivity extends AppCompatActivity {
private boolean mIsServiceRunning = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button startServiceButton = findViewById(R.id.start_service_button);
startServiceButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!mIsServiceRunning) {
// 启动Service
Intent intent = new Intent(MainActivity.this, SlideMenuService.class);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
// Android 8.0及以上版本需要使用startForeground方法将Service变成前台服务
startForegroundService(intent);
} else {
startService(intent);
}
mIsServiceRunning = true;
startServiceButton.setText(R.string.stop_service);
} else {
// 停止Service
Intent intent = new Intent(MainActivity.this, SlideMenuService.class);
stopService(intent);
mIsServiceRunning = false;
startServiceButton.setText(R.string.start_service);
}
}
});
}
}
```
阅读全文
相关推荐
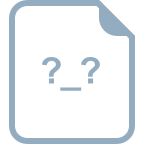
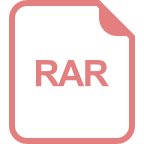
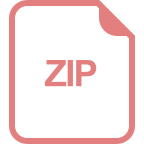
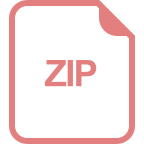
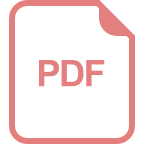
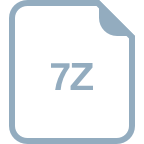
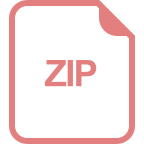
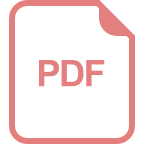
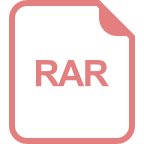
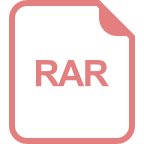
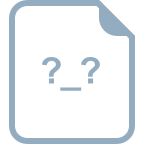
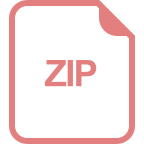
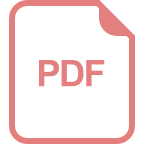
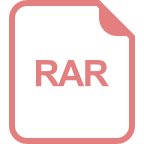
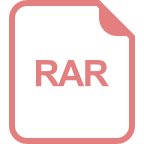
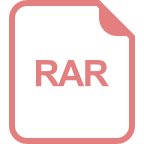