opencv人脸识别c++代码yolo模型
时间: 2023-07-24 19:56:07 浏览: 86
当您使用OpenCV进行人脸识别时,可以结合使用YOLO模型来检测人脸。下面是一个使用OpenCV和YOLO模型进行人脸识别的C++代码示例:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/dnn.hpp>
#include <iostream>
using namespace cv;
using namespace dnn;
using namespace std;
int main()
{
// 加载YOLO模型
String modelWeights = "path/to/your/yolov3.weights";
String modelConfiguration = "path/to/your/yolov3.cfg";
Net net = readNetFromDarknet(modelConfiguration, modelWeights);
// 加载图像
Mat image = imread("path/to/your/image.jpg");
if (image.empty())
{
cout << "Could not open or find the image!" << endl;
return -1;
}
// 创建一个4D blob,并将图像传递给网络
Mat blob;
double scalefactor = 1.0 / 255.0;
Size size = Size(416, 416);
Scalar mean = Scalar(0, 0, 0);
bool swapRB = true;
bool crop = false;
dnn::blobFromImage(image, blob, scalefactor, size, mean, swapRB, crop);
// 设置输入blob
net.setInput(blob);
// 运行前向传播
vector<Mat> outs;
net.forward(outs, getOutputsNames(net));
// 处理网络输出
float confThreshold = 0.5;
vector<int> classIds;
vector<float> confidences;
vector<Rect> boxes;
for (size_t i = 0; i < outs.size(); ++i)
{
// 提取每个输出层的检测结果
float* data = (float*)outs[i].data;
for (int j = 0; j < outs[i].rows; ++j, data += outs[i].cols)
{
Mat scores = outs[i].row(j).colRange(5, outs[i].cols);
Point classIdPoint;
double confidence;
minMaxLoc(scores, 0, &confidence, 0, &classIdPoint);
if (confidence > confThreshold)
{
int centerX = (int)(data[0] * image.cols);
int centerY = (int)(data[1] * image.rows);
int width = (int)(data[2] * image.cols);
int height = (int)(data[3] * image.rows);
int left = centerX - width / 2;
int top = centerY - height / 2;
classIds.push_back(classIdPoint.x);
confidences.push_back((float)confidence);
boxes.push_back(Rect(left, top, width, height));
}
}
}
// 应用非极大值抑制来删除重叠边界框
float nmsThreshold = 0.4;
vector<int> indices;
NMSBoxes(boxes, confidences, confThreshold, nmsThreshold, indices);
// 绘制人脸检测结果
for (size_t i = 0; i < indices.size(); ++i)
{
int idx = indices[i];
Rect box = boxes[idx];
rectangle(image, box, Scalar(0, 0, 255), 2);
}
// 显示结果
imshow("Face Detection", image);
waitKey(0);
return 0;
}
```
请确保您已正确安装OpenCV和YOLO模型,并将代码中的路径替换为您自己的模型和图像路径。这段代码将使用YOLO模型检测人脸,并在图像上绘制检测到的人脸边界框。
相关推荐
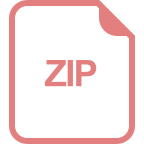
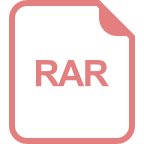
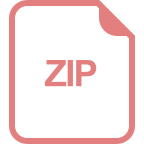














