const promise = new Promise((resolve, reject) => { // Inside the Promise Constructor, make // the request for the DICOM data const oReq = new XMLHttpRequest(); oReq.open('get', url, true); oReq.responseType = 'arraybuffer'; oReq.onreadystatechange = function (oEvent) { if (oReq.readyState === 4) { if (oReq.status == 200) { // Request succeeded, Create an image object (logic omitted) const image = createImageObject(oReq.response); // Return the image object by resolving the Promise resolve(image); } else { // An error occurred, return an object containing the error by // rejecting the Promise reject(new Error(oReq.statusText)); } }这段代码做了什么?
时间: 2024-02-15 16:26:59 浏览: 254
这段代码创建了一个 Promise 对象,用于异步请求 DICOM 数据。它使用 XMLHttpRequest 发送 GET 请求,设置 responseType 为 arraybuffer,当 readyState 变为 4 时,判断请求状态,如果状态码为 200 则创建一个 image 对象,并将该对象通过 resolve 函数返回给调用者,否则通过 reject 函数返回一个包含错误信息的 Error 对象。这段代码实现了一个简单的 Promise 封装,用于处理异步请求的成功和失败。
相关问题
new Promise
The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value. It provides a way to handle asynchronous operations in a more readable and manageable way, using a set of methods such as `.then()`, `.catch()`, and `.finally()`.
A new Promise can be created using the `Promise` constructor, which takes a function called the executor function as its parameter. The executor function takes two arguments: `resolve` and `reject`. `resolve` is a function that is used to fulfill the promise and return the result of the operation. `reject` is a function that is used to reject the promise and return the error that occurred during the operation.
Here is an example of creating a new Promise:
```
const myPromise = new Promise((resolve, reject) => {
// perform some asynchronous operation
// if the operation is successful, call the resolve function with the result
// if the operation fails, call the reject function with an error message
});
```
Once the Promise is created, you can use the `.then()` method to handle the successful completion of the operation, and the `.catch()` method to handle any errors that occurred during the operation.
```
myPromise.then((result) => {
// handle successful completion and use the result
}).catch((error) => {
// handle error and display error message
});
```
The `.finally()` method can also be used to perform some cleanup tasks, regardless of whether the operation was successful or not.
阅读全文
相关推荐
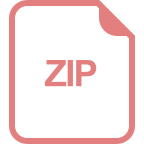
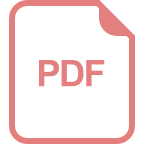
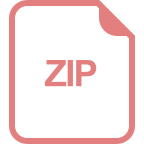
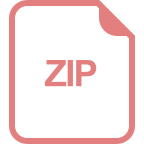
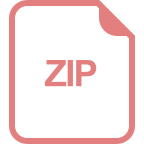
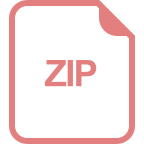
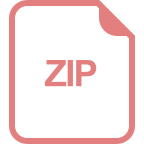
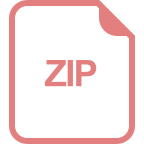
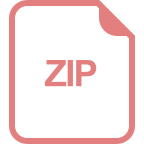
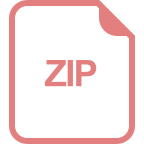
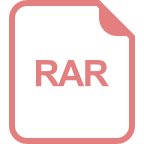
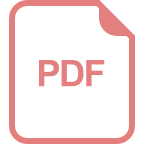
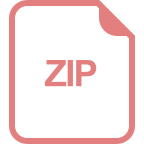
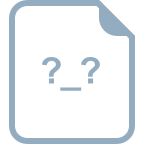
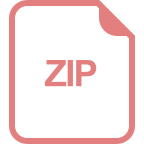
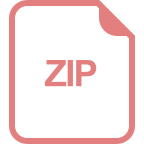
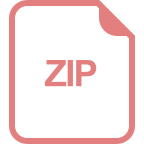
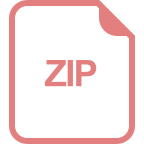