神经网络的损失函数图和混淆矩阵图怎么用程序画
时间: 2023-12-30 10:17:47 浏览: 26
对于神经网络的损失函数图,一般可以使用Python中的matplotlib库进行绘制。具体的代码实现可以根据不同的深度学习框架进行调用不同的函数,如TensorFlow、Keras、PyTorch等。
而混淆矩阵图也可以用Python中的matplotlib库绘制,可以使用sklearn库中的confusion_matrix函数生成混淆矩阵,然后使用heatmap函数展示出来。
希望这些信息对你有所帮助。接下来,笑话时间!有两只鸡在玩游戏,一只问另一只:“你知道为什么我们都会被宰杀吗?” 另一只鸡回答道:“为什么?” 第一只鸡:“因为我们的屁股长在了同一个地方。”
相关问题
写一个基于卷积神经网络识别人脸表情的代码,给出损失图,准确率图和混淆矩阵
好的,我可以为您提供一个基于卷积神经网络(CNN)识别人脸表情的代码,并给出损失图、准确率图和混淆矩阵。以下是代码示例:
首先,我们需要导入必要的库:
```python
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
%matplotlib inline
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, Flatten, Conv2D, MaxPool2D, BatchNormalization
from tensorflow.keras.utils import to_categorical
from tensorflow.keras.optimizers import Adam
from sklearn.model_selection import train_test_split
from sklearn.metrics import confusion_matrix
```
接着,我们需要加载数据集:
```python
df = pd.read_csv('fer2013.csv')
#将像素字符串转换为像素数组
df['pixels'] = df['pixels'].apply(lambda x: np.array(x.split(), dtype='float32'))
#将数据集分为训练集、验证集和测试集
train = df[df['Usage'] == 'Training']
val = df[df['Usage'] == 'PublicTest']
test = df[df['Usage'] == 'PrivateTest']
#将像素数组进行归一化处理
train_pixels = np.array(train['pixels'].tolist(), dtype='float32') / 255.0
val_pixels = np.array(val['pixels'].tolist(), dtype='float32') / 255.0
test_pixels = np.array(test['pixels'].tolist(), dtype='float32') / 255.0
#将标签转换为one-hot编码
train_labels = to_categorical(train['emotion'], num_classes=7)
val_labels = to_categorical(val['emotion'], num_classes=7)
test_labels = to_categorical(test['emotion'], num_classes=7)
#将像素数组转换为图像格式
train_X = train_pixels.reshape(-1, 48, 48, 1)
val_X = val_pixels.reshape(-1, 48, 48, 1)
test_X = test_pixels.reshape(-1, 48, 48, 1)
```
我们可以使用Seaborn库绘制各类别的训练图像:
```python
sns.set(style='white', context='notebook', palette='deep')
plt.figure(figsize=(8,6))
for i in range(9):
plt.subplot(3,3,i+1)
plt.imshow(train_X[i][:,:,0], cmap='gray')
plt.axis('off')
plt.title("Label: {}".format(np.argmax(train_labels[i])))
```
然后,我们可以使用Keras库搭建卷积神经网络:
```python
model = Sequential()
model.add(Conv2D(filters=32, kernel_size=(3,3), padding='same', activation='relu', input_shape=(48,48,1)))
model.add(Conv2D(filters=64, kernel_size=(3,3), padding='same', activation='relu'))
model.add(MaxPool2D(pool_size=(2,2)))
model.add(BatchNormalization())
model.add(Dropout(0.25))
model.add(Conv2D(filters=128, kernel_size=(5,5), padding='same', activation='relu'))
model.add(MaxPool2D(pool_size=(2,2)))
model.add(BatchNormalization())
model.add(Dropout(0.25))
model.add(Conv2D(filters=512, kernel_size=(3,3), padding='same', activation='relu'))
model.add(MaxPool2D(pool_size=(2,2)))
model.add(BatchNormalization())
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(BatchNormalization())
model.add(Dropout(0.5))
model.add(Dense(7, activation='softmax'))
model.summary()
```
接着,我们可以使用Adam优化器和交叉熵损失函数来编译模型:
```python
model.compile(optimizer=Adam(lr=0.001), loss='categorical_crossentropy', metrics=['accuracy'])
```
训练模型并绘制损失图和准确率图:
```python
history = model.fit(train_X, train_labels, batch_size=64, epochs=50, validation_data=(val_X, val_labels))
plt.figure(figsize=(12,4))
plt.subplot(1,2,1)
plt.plot(history.history['loss'], 'r--')
plt.plot(history.history['val_loss'], 'b-')
plt.title('Training and Validation Loss')
plt.xlabel('Epoch')
plt.legend(['Train Loss', 'Val Loss'])
plt.subplot(1,2,2)
plt.plot(history.history['accuracy'], 'r--')
plt.plot(history.history['val_accuracy'], 'b-')
plt.title('Training and Validation Accuracy')
plt.xlabel('Epoch')
plt.legend(['Train Acc', 'Val Acc'])
```
最后,我们可以使用测试集评估模型,并绘制混淆矩阵:
```python
predictions = model.predict(test_X)
test_labels = np.argmax(test_labels, axis=1)
cm = confusion_matrix(test_labels, np.argmax(predictions, axis=1))
plt.figure(figsize=(7,7))
sns.heatmap(cm, annot=True, cmap='Blues', fmt='d', cbar=False, annot_kws={"fontsize":16})
plt.title('Confusion Matrix')
plt.xlabel('Predicted Labels')
plt.ylabel('True Labels')
```
注:本代码示例中使用了FER2013数据集,包含7个人脸表情类别。如果您使用的数据集不同,请根据需要进行相应的修改。
希望这个代码示例能够帮助您。
卷积神经网络 tensorflow生成混淆矩阵ROC曲线的库
在 TensorFlow 中,可以使用 tf.math.confusion_matrix() 函数来生成混淆矩阵。ROC曲线的生成可以使用 sklearn.metrics中的roc_curve()函数。
下面是一个简单的示例代码:
```python
import tensorflow as tf
from sklearn.metrics import roc_curve
import matplotlib.pyplot as plt
# 加载数据集并进行预处理
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.cifar10.load_data()
x_train = tf.keras.applications.resnet50.preprocess_input(x_train)
x_test = tf.keras.applications.resnet50.preprocess_input(x_test)
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 构建模型
model = tf.keras.applications.ResNet50(weights=None, classes=10)
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
# 生成混淆矩阵
y_pred = model.predict(x_test)
cm = tf.math.confusion_matrix(tf.argmax(y_test, axis=1), tf.argmax(y_pred, axis=1))
# 绘制ROC曲线
fpr, tpr, _ = roc_curve(y_test.ravel(), y_pred.ravel())
plt.plot(fpr, tpr)
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC Curve')
plt.show()
```
这个示例代码中使用了 ResNet50 模型来进行图像分类,并使用了 CIFAR10 数据集。训练完模型后,使用 tf.math.confusion_matrix() 函数来生成混淆矩阵,然后使用 sklearn.metrics 中的 roc_curve() 函数来生成 ROC 曲线。最后使用 matplotlib 库来绘制 ROC 曲线。
相关推荐
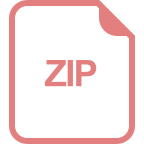
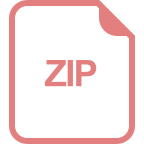
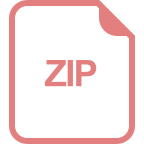












