锦标赛排序c++不要用vector库函数
时间: 2023-12-22 16:29:23 浏览: 134
锦标赛排序是一种常见的排序算法,它可以在不使用vector库函数的情况下实现。下面是一个使用数组实现锦标赛排序的C++代码示例:
```cpp
#include <iostream>
using namespace std;
// 比较两个元素的大小
int compare(int a, int b) {
return a - b;
}
// 构建锦标赛树
void buildTournamentTree(int arr[], int tree[], int n) {
for (int i = 0; i < n; i++) {
tree[n + i] = arr[i];
}
for (int i = n - 1; i > 0; i--) {
tree[i] = compare(tree[2 * i], tree[2 * i + 1]) > 0 ? tree[2 * i] : tree[2 * i + 1];
}
}
// 锦标赛排序
void tournamentSort(int arr[], int n) {
int tree[2 * n];
buildTournamentTree(arr, tree, n);
for (int i = 0; i < n; i++) {
arr[i] = tree[1];
int j = 1;
while (j < n) {
if (tree[j] == arr[i]) {
tree[j] = INT_MIN;
break;
}
j = tree[2 * j] == arr[i] ? 2 * j : 2 * j + 1;
}
while (j > 1) {
j /= 2;
tree[j] = compare(tree[2 * j], tree[2 * j + 1]) > 0 ? tree[2 * j] : tree[2 * j + 1];
}
}
}
int main() {
int arr[] = {5, 2, 8, 3, 1};
int n = sizeof(arr) / sizeof(arr[0]);
tournamentSort(arr, n);
cout << "Sorted array: ";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐









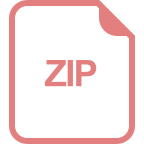


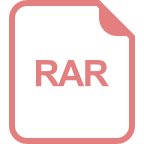
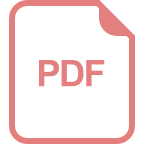

