java把foreach遍历出来的数据存入到map中
时间: 2024-05-08 17:17:09 浏览: 141
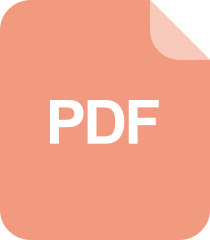
JS中Map和ForEach的区别
可以使用foreach循环遍历数组或集合,并将每个元素存储到Map中。下面是一个示例代码:
```
String[] names = {"Alice", "Bob", "Charlie"};
Map<Integer, String> map = new HashMap<>();
int index = 0;
for (String name : names) {
map.put(index, name);
index++;
}
// 输出Map中的数据
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
输出结果为:
```
0: Alice
1: Bob
2: Charlie
```
在上面的代码中,我们首先定义了一个字符串数组names和一个空的HashMap。然后,我们使用foreach循环遍历names数组,并将每个元素存储到Map中。为了保持顺序,我们使用一个整数变量index来作为键值。最后,我们使用另一个foreach循环输出Map中的数据。
阅读全文
相关推荐
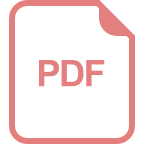
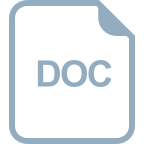
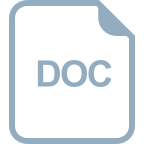
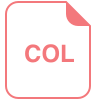
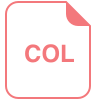











