@ControllerAdvice怎么获取request 里body的入参
时间: 2024-01-10 17:43:58 浏览: 149
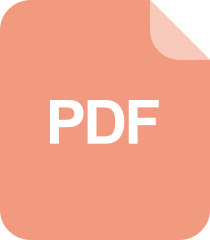
SpringMVC的Body参数拦截的问题
在 `@ControllerAdvice` 中获取 request 的 body 有多种方式,以下是其中两种常用的方式:
1. 使用 `HttpServletRequest` 对象获取请求的 body
可以使用 `HttpServletRequest` 对象获取请求的 body,然后再进行相应的处理。示例代码如下:
```java
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import javax.servlet.http.HttpServletRequest;
import java.io.BufferedReader;
import java.io.IOException;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public void handleException(HttpServletRequest request, Exception e) {
String requestBody = getRequestBody(request);
// 处理请求的 body
}
private String getRequestBody(HttpServletRequest request) {
StringBuilder requestBody = new StringBuilder();
try (BufferedReader reader = request.getReader()) {
String line;
while ((line = reader.readLine()) != null) {
requestBody.append(line);
}
} catch (IOException e) {
// 处理异常
}
return requestBody.toString();
}
}
```
2. 使用 `@RequestBody` 注解获取请求的 body
可以在 `@ControllerAdvice` 中定义一个参数为 `@RequestBody` 的入参来获取请求的 body。示例代码如下:
```java
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.context.request.ServletWebRequest;
import org.springframework.web.context.request.WebRequest;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleException(WebRequest request, @RequestBody String requestBody, Exception e) {
// 处理请求的 body
HttpHeaders headers = new HttpHeaders();
HttpStatus status = HttpStatus.INTERNAL_SERVER_ERROR;
// 构建响应体
return new ResponseEntity<>(responseBody, headers, status);
}
}
```
需要注意的是,使用第二种方式需要确保请求的 body 可以被读取,否则将会导致异常。同时,需要在 `@ControllerAdvice` 中添加 `@RestController` 注解才能正常返回响应体。
阅读全文
相关推荐
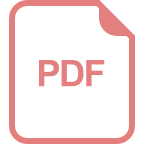
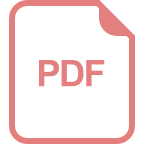


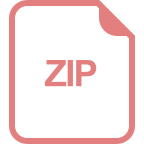
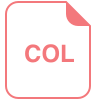
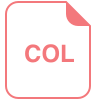
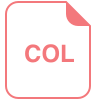







