vue3中语法糖怎么用prop接受数据
时间: 2023-12-24 17:06:14 浏览: 11
在Vue3中,使用语法糖来接受父组件传入的数据非常简单,只需要在子组件中使用`props`选项即可。
具体操作步骤如下:
1. 在子组件中使用`props`选项来声明要接受的属性。
```
<script>
export default {
name: 'ChildComponent',
props: {
message: String
}
}
</script>
```
2. 在父组件中使用子组件,并传入要接受的属性。
```
<template>
<div>
<child-component :message="'Hello, World!'"/>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
name: 'ParentComponent',
components: {
ChildComponent
}
}
</script>
```
在上面的示例中,子组件中使用了`props`选项声明了一个名为`message`的属性,类型为`String`。在父组件中使用子组件时,使用`v-bind`指令将`message`属性的值绑定为一个字符串。
这样,父组件就可以将数据传递给子组件了。
相关问题
vue3setup语法糖prop父传子
在 Vue3 的 setup 函数中,如果需要进行父组件向子组件传值,可以使用 prop 语法糖。
在子组件中,可以使用 defineProps 函数来定义需要接收的 props,如下所示:
```
import { defineProps } from 'vue'
export default {
props: {
msg: String
},
setup(props) {
// 使用 props.msg 进行操作
}
}
```
在父组件中,可以直接将需要传递的值作为子组件的属性进行传递,如下所示:
```
<template>
<ChildComponent msg="Hello World" />
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
}
}
</script>
```
这样,在子组件中,就可以通过 props.msg 来获取父组件传递的值了。如果需要进行类型检查,可以使用 defineProps 函数来定义 props 的类型和默认值。例如:
```
import { defineProps } from 'vue'
export default {
props: {
msg: String
},
setup(props) {
// 使用 props.msg 进行操作
}
}
// 使用 defineProps 进行类型检查
const props = defineProps({
msg: {
type: String,
default: 'Hello World'
}
});
```
vue3 steup语法糖
Vue3中的setup语法糖是一种简化组件编写的方式,它可以让我们在组件中直接使用Composition API的功能,而无需编写setup函数。通过在script标签中添加setup属性,我们可以直接在其中编写代码,而不需要注册属性和方法,也不需要再返回。这样,我们可以在template模板中直接使用这些属性和方法。
在使用setup语法糖后,我们可以使用一些新增的API来更方便地编写组件。其中,defineProps用于子组件接收父组件传递的props,defineEmits用于子组件调用父组件的方法,defineExpose用于子组件暴露属性,可以让父组件访问到这些属性。
举个例子,如果我们在父组件中传递一个名为numb的prop给子组件,我们可以在子组件中使用defineProps来接收这个prop,并在template中直接使用它。
```html
<template>
<my-component @click="func" :numb="numb"></my-component>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
import myComponent from '@/components/myComponent.vue';
// 使用defineProps接收父组件传递的props
const props = defineProps({
numb: Number
});
// 在template中直接使用props.numb
let func = () => {
console.log(props.numb);
}
</script>
```
这样,我们就可以更方便地使用Vue3中的setup语法糖来编写组件了。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [使用SpringBoot2和Vue3 Setup语法糖、 Composition Api开发的简洁、易用的低代码中后台解决方案](https://download.csdn.net/download/qq_41701956/87745390)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [vue3:setup语法糖](https://blog.csdn.net/seimeii/article/details/126101422)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
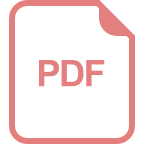
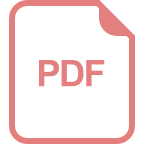
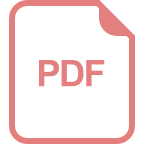













