{"name": "Tom", "age": 18} C++正则表达式取age值
时间: 2023-09-02 14:15:54 浏览: 50
可以使用C++标准库中的正则表达式模块来取出age的值。以下是一个示例代码:
```c++
#include <iostream>
#include <regex>
int main() {
std::string str = "{\"name\": \"Tom\", \"age\": 18}";
std::regex reg("\"age\":\\s*(\\d+)");
std::smatch match;
if (std::regex_search(str, match, reg)) {
std::cout << "Age: " << match[1] << std::endl;
} else {
std::cout << "Age not found" << std::endl;
}
return 0;
}
```
输出:
```
Age: 18
```
正则表达式解释:
- `\"age\":` 匹配 "\"age\":",注意需要转义双引号。
- `\\s*` 匹配零个或多个空白字符。
- `(\\d+)` 匹配一个或多个数字,并将其作为一个捕获组。
`std::regex_search()` 函数在字符串 `str` 中查找匹配正则表达式 `reg` 的子串,并将匹配的结果存储在 `match` 对象中。`match[1]` 表示第一个捕获组的值,即 age 的值。
相关问题
{"name": "Tom", "age": 18} C++正则表达式取age和name值
可以使用 C++ 的正则表达式库 `regex` 来实现,以下是示例代码:
```c++
#include <iostream>
#include <regex>
int main() {
std::string json_str = R"({"name": "Tom", "age": 18})";
std::regex age_regex(R"("age":\s*(\d+))");
std::regex name_regex(R"("name":\s*"(\w+)")");
std::smatch match;
if (std::regex_search(json_str, match, age_regex)) {
int age = std::stoi(match[1]);
std::cout << "age: " << age << std::endl;
}
if (std::regex_search(json_str, match, name_regex)) {
std::string name = match[1];
std::cout << "name: " << name << std::endl;
}
return 0;
}
```
输出结果为:
```
age: 18
name: Tom
```
{"name": "Tom", "age": 18} C++正则表达式取 同时可取name和age值
可以使用 C++ 中的正则表达式库 `std::regex` 来提取字符串中的内容。例如,对于给定的字符串 `{"name": "Tom", "age": 18}`,可以使用以下代码来提取其中的 `name` 和 `age` 值:
```c++
#include <iostream>
#include <regex>
int main() {
std::string s = R"({"name": "Tom", "age": 18})"; // 原始字符串,注意使用 R 前缀
std::regex r(R"("name": "(\w+)", "age": (\d+))"); // 正则表达式,注意使用 R 前缀
std::smatch m; // 匹配结果存储在 smatch 对象中
if (std::regex_search(s, m, r)) {
std::cout << "name: " << m[1].str() << std::endl;
std::cout << "age: " << m[2].str() << std::endl;
}
return 0;
}
```
输出:
```
name: Tom
age: 18
```
在正则表达式 `R"("name": "(\w+)", "age": (\d+))"` 中,使用了两个捕获组 `(\w+)` 和 `(\d+)` 分别匹配 `name` 和 `age` 的值。在代码中,使用 `smatch` 对象 `m` 存储匹配结果,`m[1]` 表示第一个捕获组的结果,即 `name` 的值,`m[2]` 表示第二个捕获组的结果,即 `age` 的值。
相关推荐
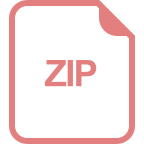
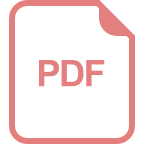
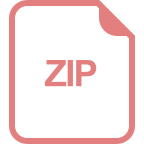












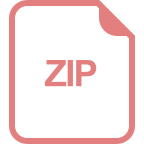