使用C语言判断网络是否畅通
时间: 2023-07-22 16:00:24 浏览: 197
要使用C语言判断网络是否畅通,可以通过发送一个简单的网络请求并等待响应来检测网络连通性。这里给出一个使用C语言的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netdb.h>
int main() {
const char* host = "www.example.com"; // 要检测的主机名
const int port = 80; // 要检测的端口号
struct sockaddr_in server;
struct hostent* hostinfo;
int sock;
// 创建套接字
sock = socket(AF_INET, SOCK_STREAM, 0);
if (sock == -1) {
printf("创建套接字失败\n");
return 1;
}
// 获取主机信息
hostinfo = gethostbyname(host);
if (hostinfo == NULL) {
printf("获取主机信息失败\n");
return 1;
}
// 设置服务器地址
server.sin_family = AF_INET;
server.sin_port = htons(port);
memcpy(&server.sin_addr, hostinfo->h_addr_list[0], hostinfo->h_length);
// 连接到服务器
if (connect(sock, (struct sockaddr*)&server, sizeof(server)) < 0) {
printf("无法连接到服务器\n");
return 1;
}
printf("网络畅通,成功连接到服务器\n");
// 关闭套接字
close(sock);
return 0;
}
```
在这个示例中,我们使用`socket()`函数创建了一个套接字,然后使用`gethostbyname()`函数获取要检测的主机的IP地址。接下来,我们设置了服务器的地址和端口号,并使用`connect()`函数尝试连接到服务器。如果连接成功,则表示网络畅通,否则表示网络不可达。最后,我们关闭了套接字。
请注意,在实际应用中,你可能需要根据自己的需求进行更复杂的网络检测,比如设置超时时间、处理错误等。以上示例仅提供了一个简单的网络连通性判断的基本框架。
阅读全文
相关推荐
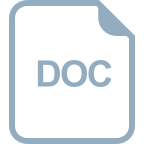
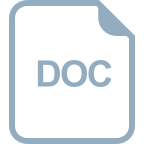
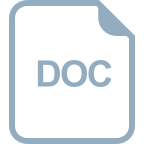
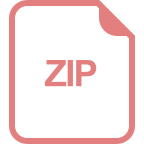
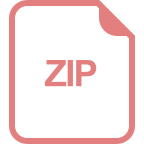
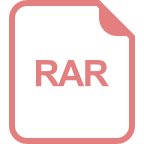
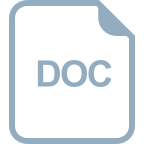
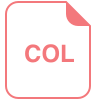
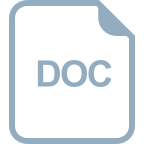
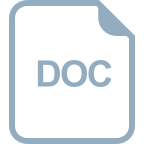
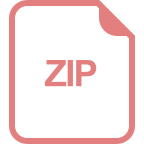
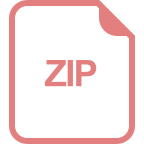
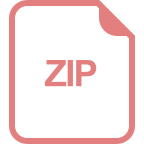
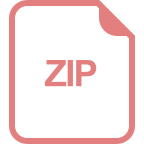