import java.util.Arrays; import java.util.Scanner; public class Main4 { public static void main(String[] args) { int[] a = {12, 54, 1, 36, 98, 11, 3, 89, 62, 7}; int i, j, temp; for (i = 0; i < a.length - 1; i++) for (j = 0; j < a.length - 1; j++) if (a[j] > a[j + 1]) { temp = a[j]; a[j] = a[j + 1]; a[j + 1] = temp; } System.out.print(Arrays.toString(a)); System.out.println(); int n = search(a); System.out.print("查询所对应的索引为" + n); } public static int search(int[] a) { int left = 0, right = a.length - 1, x; System.out.println("输入要查找的数字x:"); Scanner sc = new Scanner(System.in); x = sc.nextInt(); if (x > a.length - 1 || x < a[0]) { return -1; } while (left <= right) { int mid = (left + right) / 2; if (a[mid] < x) { left = mid + 1; } else if (a[mid] > x) { right = mid - 1; } else { return mid; } } return -1; } }这段代码有什么错误
时间: 2023-06-05 18:05:30 浏览: 50
这段代码有两个地方有错误,循环没有更新计数器和二分查找中计算中间位置的公式写错。正确的代码如下:
import java.util.Arrays;
import java.util.Scanner;
public class Main4 {
public static void main(String[] args) {
int[] a = {12, 54, 1, 36, 98, 11, 3, 89, 62, 7};
int i, j, temp;
for (i = 0; i < a.length - 1; i++) {
for (j = 0; j < a.length - 1 - i; j++) {
if (a[j] > a[j + 1]) {
temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
System.out.print(Arrays.toString(a));
System.out.println();
int n = search(a);
System.out.print("查询所对应的索引为" + n);
}
public static int search(int[] a) {
int left = 0, right = a.length - 1, x;
System.out.println("输入要查找的数字x:");
Scanner sc = new Scanner(System.in);
x = sc.nextInt();
if (x > a[right] || x < a[left]) {
return -1;
}
while (left <= right) {
int mid = left + (right - left) / 2;
if (a[mid] < x) {
left = mid + 1;
} else if (a[mid] > x) {
right = mid - 1;
} else {
return mid;
}
}
return -1;
}
}
相关推荐
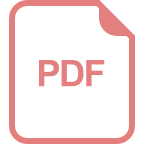
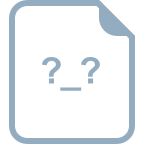
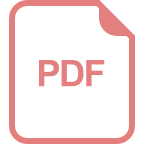
















