circle loss pytorch
时间: 2023-09-13 13:05:17 浏览: 354
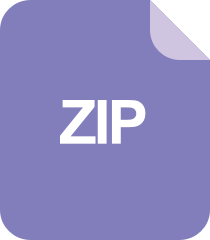
pytorch-center-loss:Pytorch实现中心损失
### 回答1:
Circle loss is a loss function used for face recognition tasks. Here is an example implementation of circle loss in PyTorch:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class CircleLoss(nn.Module):
def __init__(self, m: float, gamma: float):
super(CircleLoss, self).__init__()
self.m = m
self.gamma = gamma
def forward(self, inputs, targets):
cosine_sim = F.cosine_similarity(inputs, targets)
theta = torch.acos(torch.clamp(cosine_sim, -1, 1))
margin_cosine = torch.cos(self.m * theta)
margin_cosine -= self.gamma
one_hot = torch.zeros_like(margin_cosine)
one_hot.scatter_(1, targets.view(-1, 1).long(), 1)
output = one_hot * margin_cosine + (1 - one_hot) * cosine_sim
return output.mean()
```
In this implementation, `m` and `gamma` are hyperparameters that control the margin and scale of the loss function. `inputs` and `targets` are the embeddings and labels of the face recognition task, respectively. The loss is calculated by first computing the cosine similarity between the embeddings and the targets, then using the cosine similarity to calculate the angle `theta`. The margin cosine is then calculated using the angle and the hyperparameter `m`. Finally, the one-hot encoding of the targets is used to weight the margin cosine and the cosine similarity, and the mean is taken over the batch.
### 回答2:
Circle Loss是一种用于人脸识别任务的损失函数,它是基于深度学习框架PyTorch实现的。Circle Loss的主要目标是通过对训练样本进行特征优化,使其在特征空间中形成一个最优的球形边界。这个球形边界可以提高同类样本的相似度,并将不同类别的样本分开。
Circle Loss的优点之一是它能够捕捉到特征空间中类别之间的固有结构。与传统的softmax损失相比,Circle Loss可以更好地处理样本之间的相似度关系。它引入了一个新的超参数,即margin(边界宽度),该超参数可以调整最优球形边界的大小。
在具体实现中,Circle Loss使用了欧几里得距离度量学习来计算同类样本之间的相似度,并通过使用ArcFace角度间隔来增加不同类别样本之间的分离度。此外,Circle Loss还使用了余弦距离度量来对特征进行归一化处理,以提高优化性能。
Circle Loss可以通过编写PyTorch代码来实现。首先,需要定义一个CircleLoss的类,其中包括欧几里得距离和余弦距离的计算方法,以及损失函数的计算方法。然后,在训练过程中,将Circle Loss应用于特定的神经网络模型,在每个训练步骤中计算损失,并通过反向传播更新模型的参数。
总而言之,Circle Loss是一种用于人脸识别任务的损失函数,在PyTorch中实现。它能够通过优化样本在特征空间中的分布,提高同类样本的相似度,并将不同类别的样本分开。Circle Loss的实现主要依赖于欧几里得距离和余弦距离,并通过调整margin来调整球形边界的大小。
### 回答3:
Circle loss 是一种用于人脸识别任务的损失函数,它用于优化特征表示学习的质量,进而提高人脸识别的性能。Circle loss是在Softmax loss的基础上进行改进的。
与Softmax loss不同的是,Circle loss考虑了类间紧密性和类内分离性两个因素。它通过在特征空间中为每个类别创建一个“圆”来衡量特征之间的相似度,其中圆的半径表示类间相似性。而在圆内的样本则表示该类别的类内紧密性。
Circle loss的计算方式如下:对于每个样本,首先计算出其与其他样本之间的相似度,然后定义一个角度m,用于度量类间相似性和类内紧密性的平衡。接着,基于样本与其他类别的相似度和一组正标签(属于同一类别的样本)计算出一个动态的margin,用于调整正负样本之间的距离。
在训练过程中,Circle loss旨在最大化正样本与类内样本之间的相似度,同时最小化控制在一定范围内的负样本与类内样本之间的相似度。通过这种方式,Circle loss可以在特征空间中形成明显的类间分界,提高人脸识别的准确度和鲁棒性。
在PyTorch中,可以通过构建自定义的损失函数来实现Circle loss。首先,需要计算样本之间的相似度得分,然后根据得分计算出损失值。最后,通过反向传播和优化器更新网络参数来训练模型。
总之,Circle loss是一种用于人脸识别任务的损失函数,能够优化特征表示学习的质量,提高人脸识别的性能。在PyTorch中,可以通过自定义损失函数的方式来使用Circle loss。
阅读全文
相关推荐
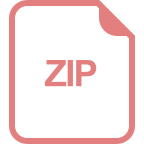




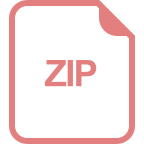
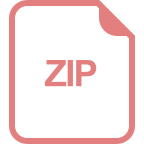
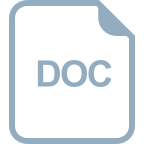


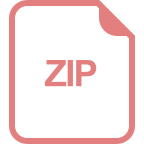
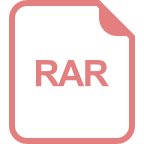
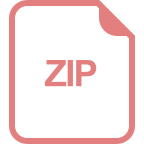
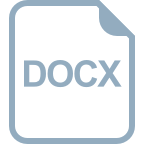
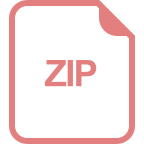