int **map;
时间: 2024-06-25 09:00:28 浏览: 4
`int **map;` 是 C 或 C++ 中的一种声明方式,它定义了一个指向指针的指针,也就是一个二维整数数组的动态存储空间。在这个表达式中:
- `int` 表示数组元素的数据类型,这里是整数。
- `**` 是两个星号,表示一个指针到指针。这意味着 `map` 可以指向一个 `int` 类型的一维数组,每个元素本身又是一个指向 `int` 的指针。
这样声明的一个常见用途是在创建或管理动态分配的二维数组,例如地图数据结构,或者在需要动态调整大小和内容的复杂数据结构中。
相关问题--
1. 如何动态地为这样的 `map` 分配内存?
2. 为什么要使用双星号(`**`)而不是单星号(`*`)?
3. 这种声明在实际编程中有哪些常见的应用场景?
相关问题
对用**map_way表示的迷宫地图,其中0为撞墙1为通路,写一个DFS(int **map_way)自动求解的C语言函数
好的,我可以为您编写一个基于深度优先搜索的迷宫求解函数。以下是代码实现:
```c
#define MAX_ROW 100
#define MAX_COL 100
int dfs(int **map_way, int row, int col, int end_row, int end_col, int **visited, int path_len, int *path) {
if (row == end_row && col == end_col) {
return path_len;
}
visited[row][col] = 1;
int next_row, next_col;
int directions[4][2] = {{-1,0}, {0,1}, {1,0}, {0,-1}};
for (int i = 0; i < 4; i++) {
next_row = row + directions[i][0];
next_col = col + directions[i][1];
if (next_row >= 0 && next_row < MAX_ROW && next_col >= 0 && next_col < MAX_COL &&
map_way[next_row][next_col] == 1 && visited[next_row][next_col] == 0) {
path[path_len] = i;
int new_path_len = dfs(map_way, next_row, next_col, end_row, end_col, visited, path_len+1, path);
if (new_path_len > 0) {
return new_path_len;
}
}
}
visited[row][col] = 0;
return 0;
}
int *maze_solver(int **map_way, int start_row, int start_col, int end_row, int end_col) {
int **visited = (int **)malloc(sizeof(int *) * MAX_ROW);
for (int i = 0; i < MAX_ROW; i++) {
visited[i] = (int *)calloc(MAX_COL, sizeof(int));
}
int *path = (int *)malloc(sizeof(int) * MAX_ROW * MAX_COL);
int path_len = dfs(map_way, start_row, start_col, end_row, end_col, visited, 0, path);
if (path_len > 0) {
int *solution = (int *)malloc(sizeof(int) * path_len);
for (int i = 0; i < path_len; i++) {
solution[i] = path[i];
}
free(path);
for (int i = 0; i < MAX_ROW; i++) {
free(visited[i]);
}
free(visited);
return solution;
} else {
free(path);
for (int i = 0; i < MAX_ROW; i++) {
free(visited[i]);
}
free(visited);
return NULL;
}
}
```
函数maze_solver接受一个指向迷宫地图的指针map_way,起点和终点行列坐标,返回一个指向解决方案的指针。解决方案是一个整型数组,每个元素表示从起点到终点的行进方向(0表示向上,1表示向右,2表示向下,3表示向左)。如果无法找到解决方案,则返回NULL。
函数内部定义了一个dfs函数,实现了深度优先搜索算法。该函数接受已访问节点的状态visited,行进过的路径path,以及当前节点的行列坐标。如果当前节点是终点,则返回路径长度;否则,对当前节点的四个相邻节点进行递归搜索,直到找到终点或者所有路径均已搜索完毕。在搜索过程中,需要检查节点是否越界、是否是墙、是否已经被访问过。
由于迷宫地图的大小是预定义的,所以函数中使用了宏定义MAX_ROW和MAX_COL来表示最大行列数。同时,为了避免栈溢出,使用了动态内存分配。函数内部动态分配了visited和path数组,需要在函数结束时手动释放。
希望这个函数对您有所帮助!
void draw_star(int **map,int *star, int height, int weight){ int sum = 0; // 空格子的个数 int index = 0; // 剩下的格子 // 算出空格子的个数 for(int i = 0; i < height ; ++i){ for(int j = 0; j < weight; ++j){ if(map[i][j] == 0) { sum += 1; } } } index = random(sum) + 1; // 在1到sum 中随机的位置生成星星 //生成星星 for(int i = 0; i < height; ++i){ for(int j = 0; j < weight; ++j){ if(map[i][j] == 0){ index -= 1; } if(index == 0){ star[0] = i; star[1] = j; return ; } } } return; } else if ( i == star[0] && j == star[1]) { cout << "★"; }这个代码使用了什么数据结构
这个代码同样使用了一个二维数组来表示游戏的地图,用于记录地图上每个位置的状态。此外,还使用了一个一维数组来记录星星的位置,其中第一个元素表示星星所在位置的行坐标,第二个元素表示星星所在位置的列坐标。这个数据结构方便对游戏的地图和星星进行维护,方便生成和更新星星的位置,并且可以方便地输出地图的状态,以便于游戏的显示和调试。
相关推荐
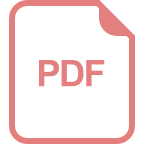
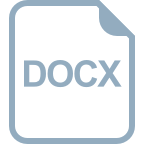












