C++ unordered_map<int, pair<int,list<int>::iterator>> cache; 如果key存在,则获取key对应的值
时间: 2024-09-09 21:15:39 浏览: 69
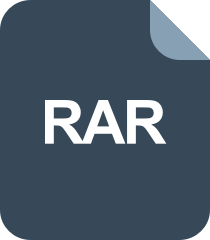
C++11 unordered_map与map(插入,遍历,Find)效率对比。
`unordered_map` 是 C++ 标准库中的一个容器,它实现了哈希表,可以提供平均常数时间复杂度的查找、插入和删除操作。在你的代码示例中,`unordered_map` 的键(key)类型为 `int`,而值(value)类型为 `pair<int, list<int>::iterator>` 的复合类型。这意味着每个键对应一个 `pair`,`pair` 的第一个元素是 `int` 类型,第二个元素是一个指向 `list<int>` 中元素的迭代器。
如果要检查键是否存在,并获取对应的值,可以使用 `unordered_map` 的 `find` 方法来查找键对应的元素。如果 `find` 方法返回的迭代器不等于 `unordered_map` 的 `end()` 方法返回的迭代器,则表示该键存在,否则不存在。
下面是一个例子:
```cpp
#include <iostream>
#include <unordered_map>
#include <list>
#include <utility> // for std::pair
int main() {
std::unordered_map<int, std::pair<int, std::list<int>::iterator>> cache;
// 假设 cache 已经填充了一些数据
int key = 10; // 我们要查找的键
// 查找键是否存在
auto it = cache.find(key);
if (it != cache.end()) {
// 键存在,获取值
std::pair<int, std::list<int>::iterator> value = it->second;
std::cout << "Key " << key << " exists with value " << value.first << " and iterator pointing to "
<< *(value.second) << std::endl;
} else {
// 键不存在
std::cout << "Key " << key << " does not exist." << std::endl;
}
return 0;
}
```
这段代码首先尝试查找键为 `10` 的元素。如果找到了,它会输出键对应的值和迭代器指向的元素;如果没有找到,则输出键不存在的信息。
阅读全文
相关推荐
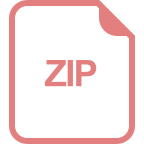
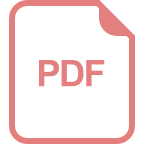
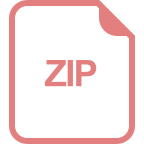
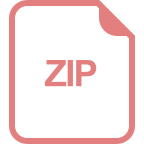
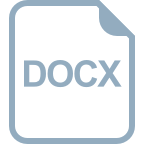
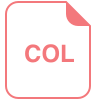
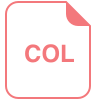
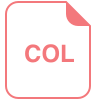
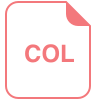








