Iterator Traversal Methods and Performance Analysis of unordered_map
发布时间: 2024-09-15 18:18:27 阅读量: 30 订阅数: 32 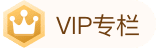
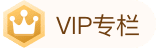
# 1. Introduction to unordered_map
**1.1 Overview of unordered_map**
The unordered_map is one of the associative containers in the C++ Standard Template Library (STL), implemented using a hash table. It provides the capability for quick look-up, insertion, and deletion of elements, with a time complexity of O(1). Compared to a map, an unordered_map does not maintain the order of elements as they are inserted; instead, it organizes data based on hash values, resulting in faster search speeds.
**1.2 Characteristics of unordered_map**
The unordered_map has the following characteristics:
- Unordered: Elements are stored in the container without any particular order.
- Fast search: Realized through a hash table, which provides efficient search capabilities.
- Dynamic growth: Supports dynamic expansion without performance degradation due to the insertion of too many elements.
- High insertion/deletion efficiency: With a time complexity of O(1).
- Does not support ordered operations: It does not offer operations to sort elements by keys. If sorting functionality is required, the map container should be used.
The introduction of unordered_map provides us with an efficient data structure for storing key-value pairs, making it an extremely practical choice for managing large volumes of data.
# 2. Basic Operations of unordered_map
- **2.1 Inserting Elements**
The unordered_map provides functionality for inserting elements into the container, allowing for the insertion of individual elements as well as multiple elements at once.
- **2.1.1 Inserting a Single Element**
To insert a single element, the `insert()` method or the `[]` operator can be used.
```cpp
// Using the insert() method to insert a single element
std::unordered_map<int, std::string> myMap;
myMap.insert({1, "apple"});
// Using the [] operator to insert a single element
myMap[2] = "banana";
```
In the above examples, the `insert()` method and the `[]` operator are used to insert individual elements into `myMap`.
- **2.1.2 Inserting Multiple Elements**
If multiple elements need to be inserted at once, the `insert()` method can be used in conjunction with an initializer list.
```cpp
// Using the insert() method to insert multiple elements
myMap.insert({{3, "cherry"}, {4, "date"}});
```
The above code uses the `insert()` method and an initializer list to insert multiple key-value pairs into `myMap` at once.
- **2.2 Deleting Elements**
Within an unordered_map, elements can be deleted by key, including the deletion of specific elements and clearing the entire unordered_map.
- **2.2.1 Deleting a Specific Element**
The `erase()` method can be used to delete the element corresponding to a given key.
```cpp
// Deleting the element corresponding to a specific key
myMap.erase(3);
```
The above code deletes the element with the key value of 3.
- **2.2.2 Clearing the Entire unordered_map**
To clear an entire unordered_map, the `clear()` method is used.
```cpp
// Clearing the entire unordered_map
myMap.clear();
```
The above code clears the entire `myMap`.
With these operations, inserting and deleting elements from an unordered_map becomes a convenient and flexible task.
# 3. Using Iterators with unordered_map
#### 3.1 Concept of Iterators
An iterator is an abstract
0
0
相关推荐








