Thread Safety Analysis of unordered_map and Multithreading Usage Tips
发布时间: 2024-09-15 18:24:45 阅读量: 30 订阅数: 26 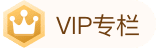
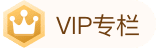
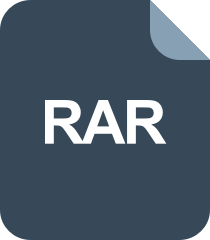
C++11 unordered_map与map(插入,遍历,Find)效率对比。
# 1. Introduction
In modern software development, utilizing an unordered_map is a highly efficient data structure that offers rapid lookup and insertion operations, making it suitable for a variety of business scenarios. However, with the prevalence of multicore processors, multithreaded programming has become increasingly important. Multithreaded programming can fully utilize hardware resources, enhancing the performance and concurrency capabilities of programs. This chapter will delve into the thread safety issues of unordered_map in a multithreaded environment, analyze the challenges that may arise when using unordered_map in a concurrent context, and discuss potential solutions. Additionally, we will introduce how to use unordered_map in a multithreaded setting and share tips and experiences to help developers better understand and apply unordered_map.
# 2. Analysis of Thread Safety for unordered_map
### 2.1 Basic Characteristics of unordered_map
#### 2.1.1 Principles and Implementation of unordered_map
The unordered_map is an associative container in the C++ standard library that uses a hash table as its internal mechanism. The hash table maps keys to buckets through a hash function, enabling rapid lookup, insertion, and deletion operations.
#### 2.1.2 Basic Operations of unordered_map
The unordered_map provides common operations such as insertion, lookup, and deletion. During insertion, the hash value is computed to find the corresponding bucket, where the key-value pair is then added. For lookup, the hash value is used to determine the bucket location, and then the target element is searched for within the bucket.
### 2.2 Challenges with unordered_map in a Multithreaded Environment
#### 2.2.1 Data Rivalry Issues
In a multithreaded environment, simultaneous insertion, lookup, and deletion operations can lead to data rivalry issues, compromising the internal consistency of the unordered_map.
#### 2.2.2 Access Conflicts and Thread Safety
When multiple threads operate on an unordered_map concurrently, it can result in access conflicts, leading to data loss or inconsistency. Therefore, ensuring the thread safety of unordered_map in a multithreaded environment is crucial.
#### 2.2.3 Discussion of Solutions
To address the thread safety issues of unordered_map in a multithreaded environment, common methods include the use of mutexes, lock granularity optimization, and lock-free data structures. These methods have their pros and cons in practical applications and require choosing the appropriate solution based on the specific scenario.
# 3. Tips for Using unordered_map in a Multithreaded Environment
When using `unordered_map` in a multithreaded environment, considerations for thread safety and performance optimization are necessary. This chapter will introduce how to ensure the correctness and efficiency of `unordered_map` in a multithreaded context by employing techniques such as mutexes, lock granularity optimization, and lock-free data structures.
#### 3.1 Protecting `unordered_map` with Mutexes
##### 3.1.1 Introduction to Mutexes
A mutex is a synchronization primitive used to protect critical sections, ensuring that only one thread can access critical resources at any given time. In C++, `std::mutex` can be used to implement mutexes.
##### 3.1.2 Applying Mutexes on `unordered_map`
By locking the mutex before operating on the `unordered_map` and releasing the lock after the operation is completed, data rivalry issues due to multiple threads accessing the `unordered_map` simultaneously can be effectively avoided.
```cpp
#include <mutex>
#include <unordered_map>
std::unordered_map<int, std::string> myMap;
std::mutex mtx;
void insertToMap(int key, const std::string& value) {
std::lock_guard<std::mutex> lock(mtx);
myMap[key
```
0
0
相关推荐
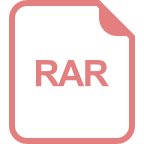
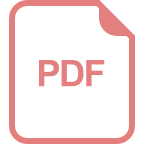
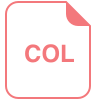
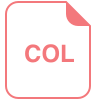
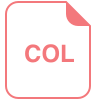
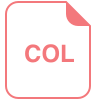
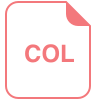
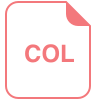