In-depth Explanation of Initialization and Assignment Methods for unordered_map
发布时间: 2024-09-15 18:16:50 阅读量: 26 订阅数: 33 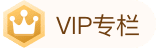
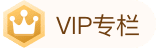
# 1. Introduction to unordered_map
The `unordered_map` is an associative container in the C++ Standard Template Library (STL) that internally uses a hash table, ensuring that the time complexity for inserting, deleting, and finding elements is O(1). It provides the ability for rapid lookups, making it more efficient than a regular `map` when searching for elements.
The `unordered_map` supports fast insertions and deletions and offers higher efficiency in finding elements because it uses a hash table internally, allowing for direct access to the position of the corresponding element via a hash function. When quick element lookup is needed without the requirement for ordered arrangement, `unordered_map` is an excellent choice.
The template declaration for `unordered_map` is `std::unordered_map<key_type, value_type>`, where `key_type` is the type of the key and `value_type` is the type of the value. Through key-value pair storage, it enables convenient and quick retrieval of values.
# 2. Initializing an unordered_map
The `unordered_map` is an associative container within the C++ STL that employs a hash table to facilitate rapid insertion, deletion, and lookup operations. Before using an `unordered_map`, it must be initialized. This chapter will introduce three methods for initializing an `unordered_map` and will demonstrate the use of each method with specific examples.
#### 2.1 Initializing an unordered_map with curly braces
Initializing an `unordered_map` with curly braces is a straightforward and convenient approach that allows for initialization by directly specifying key-value pairs. The example code is as follows:
```cpp
// Initializing an empty unordered_map
unordered_map<int, string> my_map1;
// Initializing an unordered_map with key-value pairs
unordered_map<int, string> my_map2 = {{1, "apple"}, {2, "banana"}, {3, "orange"}};
```
#### 2.2 Initializing an unordered_map with make_pair
We can also utilize the `make_pair` function to create key-value pairs, which are then inserted into the `unordered_map` for initialization. The example code is as follows:
```cpp
// Initializing an empty unordered_map
unordered_map<int, string> my_map3;
// Inserting key-value pairs for initialization
my_map3.insert(make_pair(1, "apple"));
my_map3.insert(make_pair(2, "banana"));
my_map3.insert(make_pair(3, "orange"));
```
#### 2.3 Initializing an unordered_map with the insert method
In addition to directly inserting key-value pairs with `make_pair`, we can also use the `insert` method to add elements to the `unordered_map`. The example code is as follows:
```cpp
// Initializing an empty unordered_map
unordered_map<int, string> my_map4;
// Initializing with the insert method
my_map4.insert(pair<int, string>(1, "apple"));
my_map4.insert(pair<int, string>(2, "banana"));
my_map4.insert(pair<int, string>(3, "orange"));
```
With these methods, we can flexibly initialize an `unordered_map` and choose the most suitable initialization approach based on our needs.
# 3. Insertion and Access Operations of unordered_map
#### 3.1 Inserting key-value pairs into an unordered_map
When inserting key-value pairs into an `unordered_map`, you can use the `insert` function or the subscript operator `[]`. To use the `insert` function, you need to pass a `pair` type key-value pair as a parameter. When using the subscript operator directly, if the specified key does not exist, a new key-value pair will be automatically created.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> umap;
// Inserting key-value pairs with the insert function
umap.insert(std::make_pair("apple", 10));
// Inserting key-value pairs with the subscript operator
umap["banana"] = 20;
return 0;
}
```
#### 3.2 Modifying values in an unordered_map
To modify the value associated with an existing key in an `unordered_map`, you can directly use the subscript operator `[]` or the `insert_or_assign` function. Using the subscript operator directly, if the key does not exist, an insertion operation will be performed before modifying the value; the `insert_or_assign` function, however, can directly modify the value associated with an existing key.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> umap = {{"apple", 10}, {"banana", 20}};
// Modifying a value
umap["banana"] = 30;
// Using insert_or_assign
umap.insert_or_assign("apple", 15);
return 0;
}
```
#### 3.3 Finding elements in an unordered_map
To find elements in an `unordered_map`, you can use the `find` function. If the specified key is found, it returns an iterator pointing to the key-value pair; if not found, it returns `end()`. Additionally, the `count` function can determine if a key exists, returning 1 if the key exists and 0 if it does not.
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> umap = {{"apple", 10}, {"banana", 20}};
// Finding an element
auto it = umap.find("apple");
if (it != umap.end()) {
std::cout << "Found: " << it->second << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
// Checking if a key exists
if (umap.count("banana")) {
std::cout << "Key exists" << std::endl;
} else {
std::cout << "Key does not exist" << std::endl;
}
return 0;
}
```
# 4. Traversing and Deleting Elements in an unordered_map
The `unordered_map` offers various methods for traversing and deleting elements, let's learn how to perform these operations one by one.
#### 4.1 Traversing all elements in an unordered_map
In C++, we can use iterators or range-based for loops to traverse all elements in an `unordered_map`.
##### 4.1.1 Using iterators for traversal
By using iterators to traverse an `unordered_map`, we can access each key-value pair and perform corresponding operations. Here is an example code:
```cpp
unordered_map<string, int> myMap = {{"apple", 5}, {"banana", 3}, {"cherry", 8}};
// Using iterators for traversal
for(auto it = myMap.begin(); it != myMap.end(); ++it) {
cout << "Key: " << it->first << ", Value: " << it->second << endl;
}
```
##### 4.1.2 Using range-based for loop for traversal
The range-based for loop (range-for loop) provides a more concise way to traverse an `unordered_map`:
```cpp
for(const auto& pair : myMap) {
cout << "Key: " << pair.first << ", Value: " << pair.second << endl;
}
```
#### 4.2 Deleting elements in an unordered_map
In an `unordered_map`, we can delete individual elements or clear the entire container.
##### 4.2.1 Deleting a single element
To delete a single element, use the `erase()` method, specifying the key value to delete the targeted element. The example is as follows:
```cpp
// Deleting the element with the key "banana"
myMap.erase("banana");
```
##### 4.2.2 Clearing an unordered_map
To clear the entire `unordered_map`, use the `clear()` method:
```cpp
myMap.clear();
```
With these methods, we can conveniently traverse and delete elements from an `unordered_map`, applying them flexibly in practical development.
# 5. Summary and Extensions
The `unordered_map` is an associative container provided by the C++ STL, characterized by its fast lookup, insertion, and deletion operations. This section will compare `unordered_map` with `map`, introduce additional methods for operating with `unordered_map`, and analyze its use cases.
#### 5.1 Comparison between unordered_map and map
When choosing containers from the STL's associative containers, it is often necessary to select the appropriate type based on the specific scenario. Both `unordered_map` and `map` can store key-value pairs and perform fast lookups, but their internal implementations differ.
| Feature | unordered_map | map |
|-------------------|----------------------------------|----------------------------------|
| Internal Implementation | Hash Table | Red-Black Tree |
| Lookup Efficiency | Average O(1), Worst O(n) | O(log n) |
| Orderliness | Unordered | Ordered |
| Memory Usage | Uses More Memory Space | Uses Less Memory Space |
| Suitable Scenarios| Frequent lookups, No need for ordered output | Requires ordered traversal or output, or limited memory space |
In practical applications, if the ordering of elements is not a significant concern and fast lookups and insertions are required, `unordered_map` is the preferred choice. On the other hand, if ordered traversal or output by key size is needed, or memory space is limited, `map` is the better option.
#### 5.2 Introduction to More Operations of unordered_map
In addition to insertion, access, and deletion operations, `unordered_map` also provides some other commonly used methods, such as:
- `count(key)`: Returns the number of elements in the container with the key value as key, typically used to check if an element exists.
- `size()`: Returns the number of elements in the container.
- `bucket_count()`: Returns the number of buckets in the hash table.
- `bucket(key)`: Returns the index of the bucket containing the specific key key.
- `empty()`: Determines whether the container is empty.
Here is a simple example code:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "orange"}};
// Checking if an element exists
if (myMap.count(2) > 0) {
std::cout << "Key 2 exists in the map." << std::endl;
}
// Printing the size of the map and the number of buckets
std::cout << "Size of the map: " << myMap.size() << std::endl;
std::cout << "Number of buckets: " << myMap.bucket_count() << std::endl;
return 0;
}
```
#### 5.3 Analysis of unordered_map Use Cases
Due to its high lookup efficiency, rapid insertion, and deletion operations, `unordered_map` has a wide range of practical use cases, such as:
- Caching systems: Used to store key-value pairs for faster data access.
- String processing: To count the occurrences of characters, and for quick lookup, replace operations.
- Data processing: For indexing data and rapid retrieval of specific elements.
In summary, `unordered_map` is suitable for scenarios requiring rapid lookups and insertions, especially for the storage and management of large volumes of data. When choosing a container type, it is essential to consider the specific requirements comprehensively before deciding on the use of `unordered_map`.
0
0
相关推荐







