Common Tips and Performance Optimization for Element Lookup in Unordered_MAP
发布时间: 2024-09-15 18:19:22 阅读量: 27 订阅数: 26 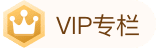
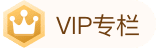
# 1. Basic Concepts and Principles of `unordered_map`
`unordered_map` is an associative container in the C++ Standard Template Library (STL), implemented using a hash table as its underlying data structure. It is used to store key-value pairs, offering fast insertion, lookup, and deletion operations. In contrast to `map`, `unordered_map` does not automatically sort the keys by their values, but instead, it maps keys to their storage positions through a hash function. This design ensures that the time complexity for insertion and lookup is constant time, making it suitable for efficient operations with large datasets. `unordered_map` excels when handling massive amounts of data, but performance may be affected by hash collisions, so it's essential to choose a suitable hash function and resolve collisions promptly. To fully leverage the advantages of `unordered_map`, developers need to have an in-depth understanding of its internal mechanisms and appropriate usage scenarios.
# 2. Inserting Elements into an Unordered Map
Inserting elements is one of the common operations when using `unordered_map`. Understanding the techniques and methods for inserting elements is crucial for improving the efficiency and performance of your code. This chapter will delve into the quick insertion tricks for elements in an unordered map and how to select the appropriate insertion methods.
#### Quick Insertion Tricks for Unordered Map Elements
The `unordered_map` supports various methods for inserting elements, with the most common being the `insert` function. The `insert` function can be used to insert either a single element or multiple elements at once, and it guarantees that the insertion process will not overwrite existing elements. Here is an example code snippet demonstrating the use of the `insert` function:
```cpp
// Define an unordered_map
unordered_map<string, int> myMap;
// Insert a single element
myMap.insert(make_pair("apple", 5));
// Insert multiple elements
myMap.insert({{"banana", 3}, {"orange", 7}});
```
In addition to the `insert` function, the `emplace` function can be used for rapid insertion. Unlike the `insert` function, `emplace` constructs elements directly using the constructor without creating temporary objects. This avoids additional copying and moving operations, enhancing insertion efficiency. Here is an example code snippet showing the use of the `emplace` function:
```cpp
// Use emplace function to insert an element
myMap.emplace("grape", 9);
```
#### How to Choose the Appropriate Insertion Method
When selecting an insertion method, it is generally best to decide based on the specific scenario. If you are certain that the elements to be inserted will not have duplicate keys, it is recommended to use the `emplace` function to avoid unnecessary construction and copying overhead. If you need to insert multiple elements or check for the presence of elements, the `insert` function might be the better choice. For a small number of elements, you can directly use `insert` or `emplace` functions; however, if you need to insert a large number of elements, consider using the automatic resizing mechanism of `unordered_map` to enhance efficiency.
In summary, in practical programming, flexibly choosing the appropriate insertion method based on the specific situation can improve the efficiency and performance of your code and ensure the normal operat
0
0
相关推荐
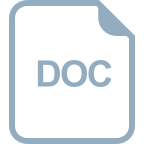
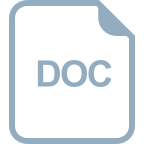
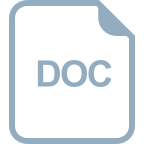
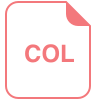
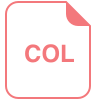
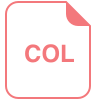
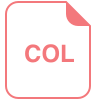
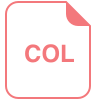
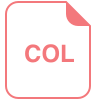