Analysis of Differences and Usage Scenarios Between unordered_map and map
发布时间: 2024-09-15 18:15:53 阅读量: 30 订阅数: 26 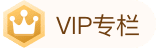
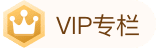
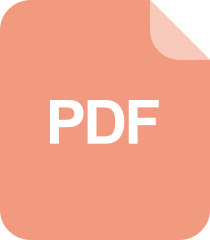
Analysis of the Differences between English and Chinese Language Structure.pdf
# 1. Understanding the Concept of Containers in STL
STL, which stands for Standard Template Library, is a significant component of the C++ programming language. Within STL, a container is a data structure designed to hold data, capable of storing various types of data and embodying principles of object-oriented programming such as encapsulation, inheritance, and polymorphism. Containers can be categorized into different types based on their internal mechanisms and functionalities, including sequential and associative containers. The vector is a commonly used sequential container in STL with the characteristics of a dynamic array, whereas the list is a doubly linked list structure, suitable for frequent insertions and deletions. By studying the various types of containers in STL, developers can handle a wide array of data structures and algorithmic problems more efficiently, enhancing the maintainability and readability of their code.
# 2. The Principle and Usage of map
### 2.1 The Underlying Mechanism of map
#### 2.1.1 Introduction to Red-Black Trees
Before delving into the mechanics of map, let's first understand the concept of red-black trees. A red-black tree is a self-balancing binary search tree that tags each node with a color attribute, which can be either red or black. Certain rules are applied to maintain this color balance, ensuring the depth of the tree remains optimal. Consequently, operations such as insertion, deletion, and search all have a time complexity of O(log n).
#### 2.1.2 The Implementation Principle of map
The map is an associative container based on the red-black tree. It stores key-value pairs in the tree, maintaining a certain order. When inserting a new element, the map automatically adjusts the structure of the red-black tree according to the key values, ensuring the tree remains balanced. This allows the map to perform lookups, insertions, and deletions swiftly, while keeping the elements ordered.
### 2.2 Basic Operations of map
#### 2.2.1 Insertion and Deletion Operations
The map provides methods such as insert and erase for element insertion and deletion. When inserting a new element, the map places it in the appropriate position based on the key value and maintains the tree's balance; upon deletion, it readjusts the tree's structure to preserve the balance.
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// Insert elements
myMap.insert(std::make_pair(1, "One"));
myMap.insert(std::make_pair(2, "Two"));
// Delete an element
myMap.erase(1);
return 0;
}
```
#### 2.2.2 Search and Modification Operations
The find method of map allows for a quick search for the value corresponding to a specified key. If found, it returns an iterator to that element; otherwise, it returns an iterator pointing to the end. By modifying the value associated with a key, one can perform element modification operations.
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
// Search for an element
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Key 2 found, value: " << it->second << std::endl;
}
// Modify an element
myMap[2] = "New Value";
return 0;
}
```
#### 2.2.3 Traversal and Iteration Operations
The map provides iterators for traversing its elements. These iterators can be incremented, decremented, and used to iterate over the elements within the map.
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
// Traverse elements
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
Through these operations, we can gain a deeper understanding of the map, an associative container based on the red-black tree, grasp its underlying mechanisms, and learn how to perform insertion, deletion, searching, and traversal.
# 3. Internal Implementation and Performance Comparison of unordered_map
### 3.1 The Hash Table Principle of unordered_map
A hash table is a data structure that allows direct access to the memory storage location using a key. The unordered_map is implemented based on hash tables. When inserting and retrieving elements, the unordered_map first computes the hash value of the element using a hash function and then locates the corresponding storage position based on this hash value.
#### 3.1.1 The Role of Hash Functions
A hash function is a technique that converts input data of arbitrary length into a fixed-length output. It maps the element's key to a definite storage position, enabling quick lookups or insertions. A good hash function minimizes collisions and improves the performance of unordered_map.
#### 3.1.2 Methods for Collision Resolution
A collision occurs when different elements, after being processed by a hash function, are mapped to the same storage location. The unordered_map typically uses separate chaining to resolve collisions, which involves storing colliding elements in data structures such as linked lists at the same storage location.
#### 3.1.3 The Resizing Mechanism of Hash Tables
When the number of elements in an unordered_map reaches a certain threshold, to avoid hash collisions and enhance efficiency, the system triggers a hash table resize, which means reallocating larger storage space and recalculating the hash values for all existing elements. This process might incur some performance overhead.
### 3.2 Performance Comparison between unordered_map and map
The unordered_map is based on hash tables, while map uses red-black trees as its underlying data structure. Their performance varies for different operations, and a detailed comparison follows.
#### 3.2.1 Time Complexity Comparison
For insertion, deletion, and search operations, the average time complexity of unordered_map is O(1), whereas for map, it is O(log n). This means that in most cases, unordered_map can complete the related operations faster than map.
#### 3.2.2 Memory Usage Comparison
Due to the implementation of hash tables, the memory footprint of unordered_map is typically larger than that of map. This is because unordered_map needs to maintain the buckets and chains of the hash table, while map only needs to maintain the nodes of the red-black tree.
#### 3.2.3 Selection of Practical Scenarios
unordered_map is suitable for scenarios requiring fast lookups, insertions, and deletions of elements, especially when the order of elements is not a concern. map, on the other hand, is suitable for scenarios involving frequent lookups on ordered data or when insertions and deletions are infrequent. When choosing a container, it's necessary to consider the actual requirements and characteristics to decide which type to use.
The above section provides a detailed introduction to the internal implementation and performance comparison of unordered_map, aiming to help you better understand the characteristics and applicable scenarios of these two types of containers.
# 4. Analysis of Applicable Scenarios for map and unordered_map
### 4.1 Applicable Scenarios for map
#### 4.1.1 Requirement for Ordered Data Storage and Search
When there is a need for ordered storage and search based on element keys, using a map is a wise choice. map is implemented based on red-black trees, ensuring elements are stored in a sorted order by key and providing efficient search operations. For instance, sorting and storing student grades by student ID allows for quick lookups by ID.
#### 4.1.2 Infrequent Insertions and Deletions of Elements
Since the red-black tree structure within a map needs to maintain balance to preserve order, frequent insertion and deletion operations can lead to frequent tree restructurings, which can impact performance. Therefore, when the insertion and deletion of elements are infrequent, and there is more emphasis on order and search efficiency, choosing a map is judicious.
### 4.2 Applicable Scenarios for unordered_map
#### 4.2.1 Need for Fast Lookup, Insertion, and Deletion Operations
unordered_map is implemented based on hash tables, offering fast lookup, insertion, and deletion operations. In scenarios involving frequent insertion, deletion, and search operations, unordered_map outperforms map. For example, when dealing with large data sets, using unordered_map can yield better performance.
#### 4.2.2 No Particular Requirement for Data Storage Order
Unlike map, unordered_map does not require the maintenance of element storage order. It relies on the hash function to compute the hash value of keys for rapid data access. It is suitable for scenarios where the storage order is not important, but efficient search capabilities are necessary.
### Comparison Table: map vs. unordered_map
| Feature | map | unordered_map |
|------------------------|-----------------------------------------|------------------------------------------|
| Internal Implementation | Red-Black Tree | Hash Table |
| Orderliness | Ordered | Unordered |
| Insertion/Deletion Performance | Slower | Faster |
| Search Performance | Faster | Fast |
| Applicable Scenarios | Scenarios requiring ordered storage and search | Scenarios with a focus on efficient insertion and search where storage order is not a concern |
### Decision Flowchart: Choosing Between map and unordered_map
```mermaid
graph LR
A[Determine Requirement Scenario] -->|Ordered Data Storage Requirement| B(map Applicable Scenario)
A -->|Fast Operation Requirement| C(unordered_map Applicable Scenario)
B --> D(Select map)
C --> E(Select unordered_map)
```
The above analysis focuses on applicable scenarios for using map and unordered_map. By selecting the appropriate container type based on the requirements, the advantages of the containers can be better utilized.
# 5. Examples of Using unordered_map and map
In actual software development, we often need to choose the right container to store and manage data based on the specific situation. In this chapter, we will demonstrate the usage methods of unordered_map and map through concrete examples and compare their applicability in different scenarios.
### 5.1 Example: Counting the Occurrence of Words
Suppose we need to count how many times each word appears in a text segment. We can use an unordered_map to accomplish this task. Here is a C++ example code:
```cpp
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
std::string text = "Hello World Hello";
std::unordered_map<std::string, int> wordCount;
std::string word;
for (int i = 0; i < text.size(); ++i) {
if (text[i] == ' ' || i == text.size() - 1) {
if (i == text.size() - 1) {
word.push_back(text[i]);
}
wordCount[word]++;
word = "";
} else {
word.push_back(text[i]);
}
}
for (const auto& pair : wordCount) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
In this example, we use an unordered_map to count the occurrences of each word. By iterating over the text and using words as keys and their counts as values, we store them in the unordered_map, and finally, print out the frequency of each word.
### 5.2 Example: Output by Key Sorting
If we need to output key-value pairs from a map in key order, we can use a map to do so. Here is a Python example code:
```python
word_count = {
'Hello': 2,
'World': 1
}
for key in sorted(word_count.keys()):
print(key, ':', word_count[key])
```
In this example, we use a Python map to store words and their occurrences, then sort the map's keys with the sorted function, and output the key-value pairs in the sorted order.
Through these two examples, we can see the flexible application of unordered_map and map in different scenarios, helping us efficiently manage various data-related issues.
### 5.3 Performance Comparison
In the examples above, we can see that unordered_map is suitable for scenarios requiring rapid insertion, search, and deletion, while map is suitable for ordered data storage and output by key sorting. In practical applications, we can choose the appropriate container based on specific requirements to achieve optimal performance and results.
By comparing practical applications and performance tests, we can better understand the applicability of unordered_map and map in different scenarios, providing more choices and inspiration for our software development work.
The above is about the examples of using unordered_map and map and their performance comparison, hoping to help readers better understand and apply these two types of containers. In practical development, choosing the right container based on the requirements is very important. It is hoped that readers can gain insights from the content of this chapter.
0
0
相关推荐
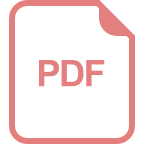
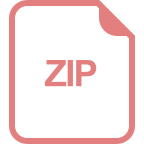
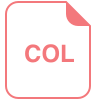
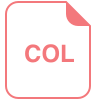
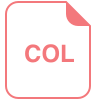
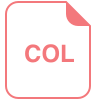
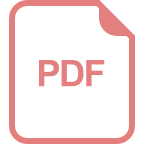
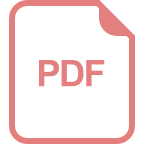
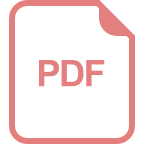