Conflict Resolution Mechanism and Solution Comparison in unordered_map
发布时间: 2024-09-15 18:23:08 阅读量: 26 订阅数: 26 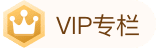
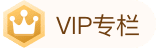
# 1. Understanding the Basics of `unordered_map`
The `unordered_map` is an associative container in the C++ ***pared to `map`, `unordered_map` employs a hash table as its underlying data structure, allowing for average time complexity of O(1) for lookup operations. Insertion and deletion of key-value pairs also exhibit high performance. The `unordered_map` uses a hash table at its core, mapping keys to their corresponding buckets via a hash function, and resolving collisions through a collision handling mechanism. In practice, `unordered_map` is well-suited for scenarios that require rapid lookup and insertion of data, without specific requirements for the order of key-value pairs. By intelligently designing hash functions and adjusting the load factor, the performance of `unordered_map` can be further optimized.
# 2. Collision Handling Mechanisms in `unordered_map`
1. Definition and Analysis of Collisions
- When using `unordered_map` to store data, it is possible for multiple distinct keys to map to the same hash bucket, resulting in a collision. This typically occurs because the range of the hash function is smaller than the range of possible key values, or different keys yield the same index position through the hash function, leading to incorrect insertion of data into the hash table.
- 1.1 Open Addressing
- Open addressing is a method to resolve hash collisions that probes for a new location sequentially when a collision occurs, continuing until an empty spot is found. This method requires that all buckets be tried to avoid data loss.
- 1.2 Chaining
- Chaining is another method to resolve hash collisions, which maintains a linked list in each bucket of the hash table. All key-value pairs that hash to the same bucket are stored in this list, ***
***mon Collision Resolution Methods in `unordered_map`
- The C++ STL implementation of `unordered_map` uses the chaining method (also known as linked address法) to handle hash collisions.
- 2.1 Linear Probing
- Linear probing is a form of open addressing that linearly probes the next position in the event of a collision, continuing until an open spot is located.
- 2.2 Double Hashing
- Double hashing is another implementation of open addressing that uses two different hash functions to calculate the step size in the probing sequence, preventing clustering of the probe sequence and improving search efficiency.
- 2.3 Chaining
- Chaining is a common method to resolve hash collisions by maintaining a linked list in each bucket of the hash table to store colliding data. When a collision occurs, the new data is inserted into the linked list of the corresponding bucket, ensuring that no data is lost. This method is simple and efficient, suitable for most situations.
# 3. Tips for Optimizing `unordered_map` Performance
1. Essentials of Hash Function Design
- 1.1 Principle of Uniform Distribution
- A hash function that distributes values uniformly can reduce collisions and improve `unordered_map` performance.
- For example, with string keys, the sum of ASCII codes of characters in the key can be used as
0
0
相关推荐
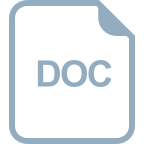
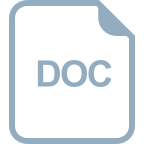
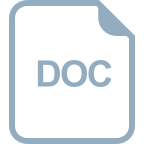
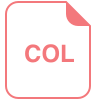
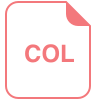
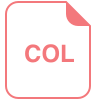
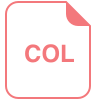
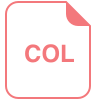
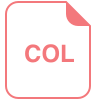